Python Exercise: Convert a given string list to a tuple
25. Convert a Given String List to a Tuple
Write a Python program to convert a given string list to a tuple.
Sample Solution:
Python Code:
# Define a function named 'string_list_to_tuple' that takes a string 'str1' as input.
def string_list_to_tuple(str1):
# Create a tuple 'result' by iterating through each character 'x' in 'str1' and excluding whitespaces.
result = tuple(x for x in str1 if not x.isspace())
# Return the resulting tuple.
return result
# Create a string 'str1' with spaces.
str1 = "python 3.0"
# Print the original string.
print("Original string:")
print(str1)
# Print the data type of the 'str1' variable.
print(type(str1))
# Print a message indicating the conversion of the string to a tuple.
print("Convert the said string to a tuple:")
# Call the 'string_list_to_tuple' function to convert the string to a tuple and print the result.
print(string_list_to_tuple(str1))
# Print the data type of the result obtained from the 'string_list_to_tuple' function.
print(type(string_list_to_tuple(str1)))
Sample Output:
Original string: python 3.0 <class 'str'> Convert the said string to a tuple: ('p', 'y', 't', 'h', 'o', 'n', '3', '.', '0') <class 'tuple'>
Flowchart:
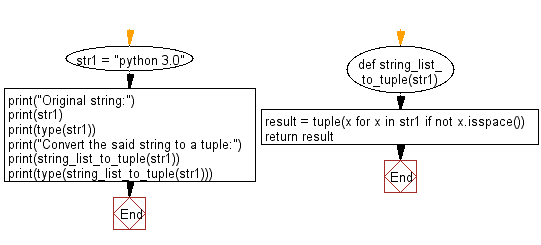
For more Practice: Solve these Related Problems:
- Write a Python program to take a space-separated string and convert it into a tuple of characters.
- Write a Python program to split a given string into words and then convert the list of words into a tuple.
- Write a Python program to implement a function that converts a string into a tuple of its individual characters using tuple().
- Write a Python program to accept user input, split it on whitespace, and output the result as a tuple.
Go to:
Previous: Write a Python program to count the elements in a list until an element is a tuple.
Next: Write a Python program calculate the product, multiplying all the numbers of a given tuple.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.