Ruby Array Exercises: Create a new array using first three elements of a given array of integers
Write a Ruby program to create a new array using first three elements of a given array of integers. If the length of the given array is less than three return the original array.
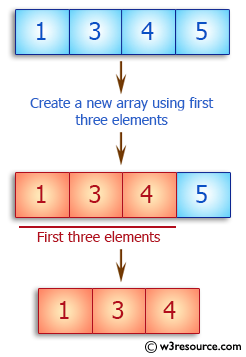
Ruby Code:
def check_array(nums)
front = []
if nums.length >= 3
front[0] = nums[0]
front[1] = nums[1]
front[2] = nums[2]
elsif nums.length == 2
front[0] = nums[0]
front[1] = nums[1]
else nums.length == 1
front[0] = nums[0]
end
return front
end
print check_array([1, 3, 4, 5]),"\n"
print check_array([1, 2, 3]),"\n"
print check_array([1,2]),"\n"
print check_array([1]),"\n"
Output:
[1, 3, 4] [1, 2, 3] [1, 2] [1]
Flowchart:
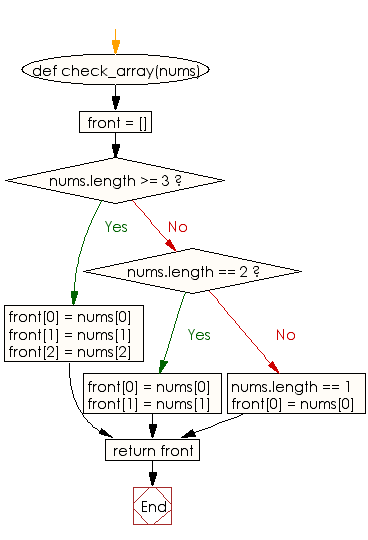
Ruby Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Ruby program to find the largest value from a given array of integers of odd length. The array length will be a least 1.
Next: Write a Ruby program to create a new array with the first element of two arrays. If lenght of any array is 0, ignore that array.
What is the difficulty level of this exercise?