SQL Exercises: Find out customers who made the order
Customer Orders Listing
From the following tables, write a SQL query to locate the orders made by customers. Return order number, customer name.
Sample table: orders
ord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample table: customer
customer_id | cust_name | city | grade | salesman_id -------------+----------------+------------+-------+------------- 3002 | Nick Rimando | New York | 100 | 5001 3007 | Brad Davis | New York | 200 | 5001 3005 | Graham Zusi | California | 200 | 5002 3008 | Julian Green | London | 300 | 5002 3004 | Fabian Johnson | Paris | 300 | 5006 3009 | Geoff Cameron | Berlin | 100 | 5003 3003 | Jozy Altidor | Moscow | 200 | 5007 3001 | Brad Guzan | London | | 5005
Sample Solution:
-- This query selects specific columns ('orders.ord_no' and 'customer.cust_name') from the 'orders' and 'customer' tables.
-- It retrieves data where the 'customer_id' column in the 'orders' table matches the 'customer_id' column in the 'customer' table.
SELECT orders.ord_no, customer.cust_name
-- Specifies the tables from which to retrieve the data (in this case, 'orders' and 'customer').
FROM orders, customer
-- Specifies the condition for joining the 'orders' and 'customer' tables based on the equality of the 'customer_id' columns.
WHERE orders.customer_id = customer.customer_id;
Output of the query:
ord_no cust_name 70009 Brad Guzan 70002 Nick Rimando 70004 Geoff Cameron 70005 Brad Davis 70008 Nick Rimando 70010 Fabian Johnson 70003 Geoff Cameron 70011 Jozy Altidor 70013 Nick Rimando 70001 Graham Zusi 70007 Graham Zusi 70012 Julian Green
Code Explanation:
The said query in SQL that joins the orders' and 'customer' tables based on the "customer_id" column. The result set includes the order number (ord_no) and customer name (cust_name). The WHERE clause specifies the join condition between the two tables, which is that the "customer_id" column must be equal in both tables.
Relational Algebra Expression:

Relational Algebra Tree:
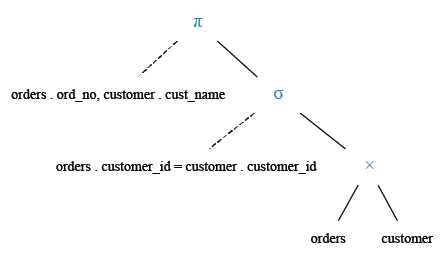
Explanation:
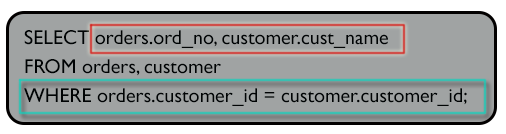
Visual presentation :
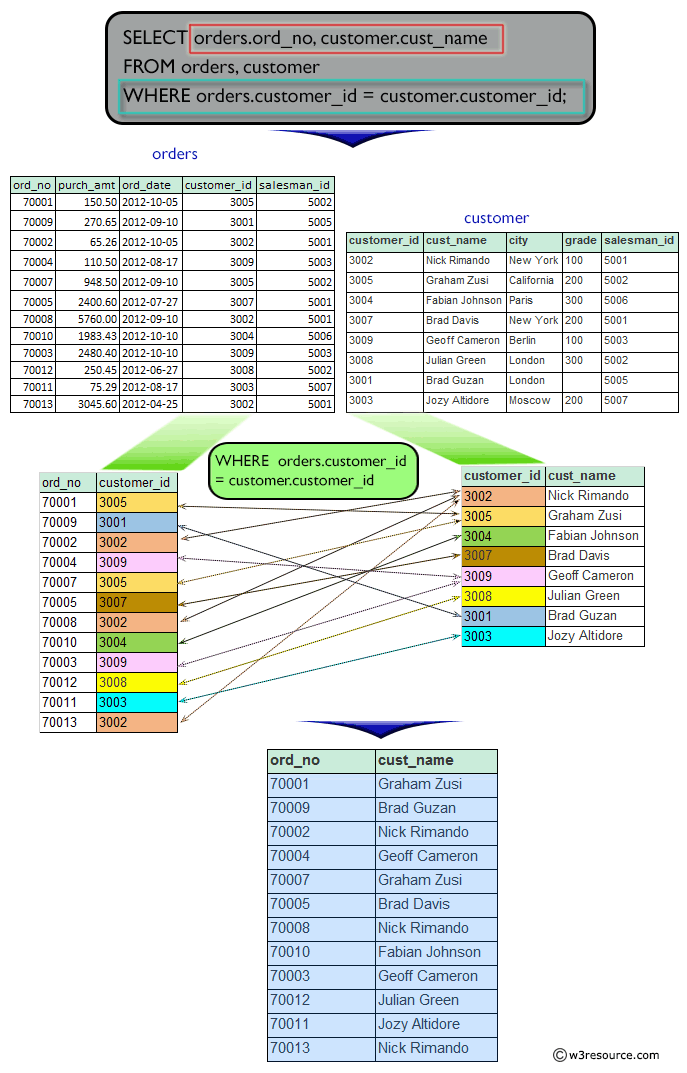
Practice Online
Query Visualization:
Duration:
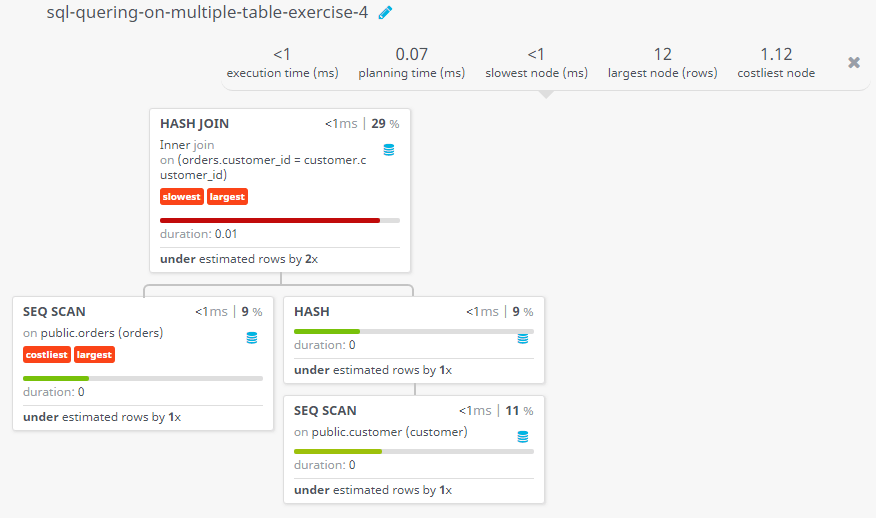
Rows:
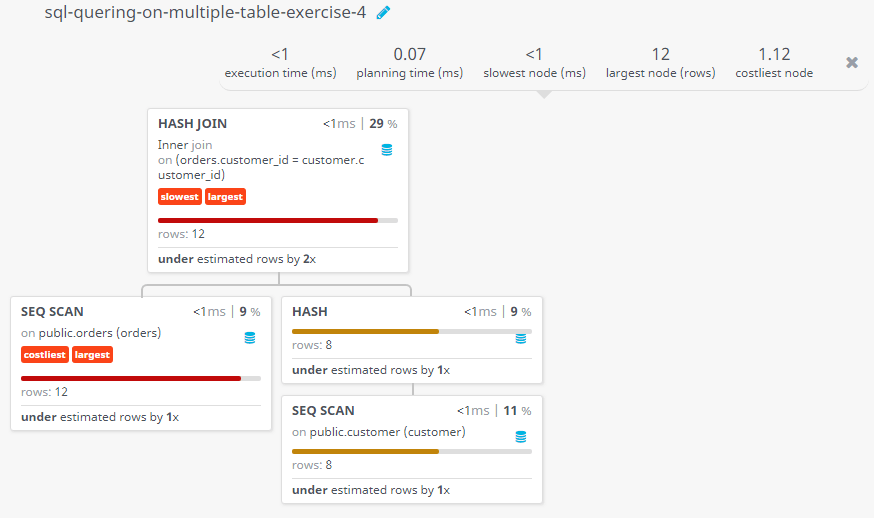
Cost:
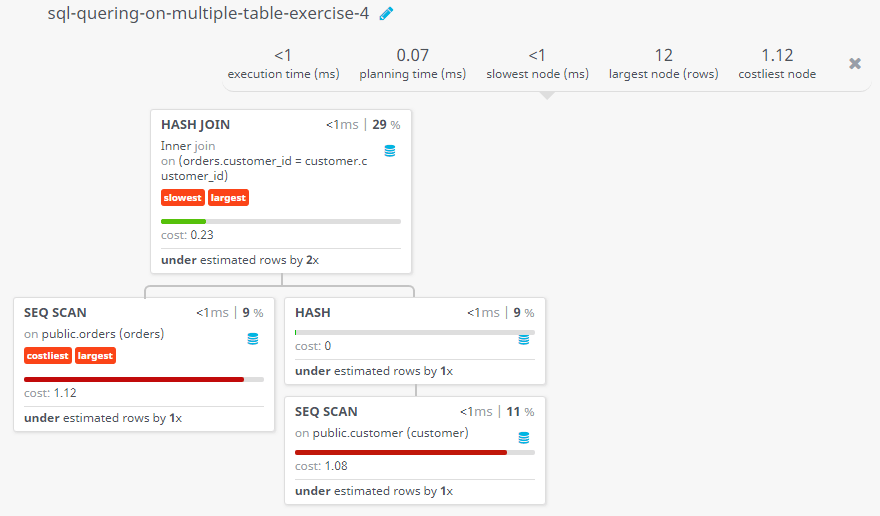
For more Practice: Solve these Related Problems:
- Write a SQL query to retrieve order numbers and customer names, including only those orders where the customer's city is not null.
- Write a SQL query to display the most recent order number for each customer along with the customer’s name.
- Write a SQL query to list order numbers with customer names for orders where the purchase amount exceeds the overall average purchase amount.
- Write a SQL query to display distinct order numbers and corresponding customer names for customers who have placed more than two orders.
- Write a SQL query to fetch order numbers and customer names, ordering the results by the order date in descending order.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Customer lives in a city other than the salesman's.
Next SQL Exercise: Sort out the customer who made at least an order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.