SQL Exercises: Sort out the customer who made at least an order
Customer Grade & Salesperson City
From the following tables, write a SQL query to find those customers where each customer has a grade and is served by a salesperson who belongs to a city. Return cust_name as "Customer", grade as "Grade".
Sample table: orders
ord_no purch_amt ord_date customer_id salesman_id ---------- ---------- ---------- ----------- ----------- 70001 150.5 2012-10-05 3005 5002 70009 270.65 2012-09-10 3001 5005 70002 65.26 2012-10-05 3002 5001 70004 110.5 2012-08-17 3009 5003 70007 948.5 2012-09-10 3005 5002 70005 2400.6 2012-07-27 3007 5001 70008 5760 2012-09-10 3002 5001 70010 1983.43 2012-10-10 3004 5006 70003 2480.4 2012-10-10 3009 5003 70012 250.45 2012-06-27 3008 5002 70011 75.29 2012-08-17 3003 5007 70013 3045.6 2012-04-25 3002 5001
Sample table: customer
customer_id | cust_name | city | grade | salesman_id -------------+----------------+------------+-------+------------- 3002 | Nick Rimando | New York | 100 | 5001 3007 | Brad Davis | New York | 200 | 5001 3005 | Graham Zusi | California | 200 | 5002 3008 | Julian Green | London | 300 | 5002 3004 | Fabian Johnson | Paris | 300 | 5006 3009 | Geoff Cameron | Berlin | 100 | 5003 3003 | Jozy Altidor | Moscow | 200 | 5007 3001 | Brad Guzan | London | | 5005
Sample Solution:
-- This query selects specific columns ('customer.cust_name' with alias "Customer", 'customer.grade' with alias "Grade", 'orders.ord_no' with alias "Order No.")
-- from the 'orders', 'salesman', and 'customer' tables.
-- It retrieves data where the 'customer_id' column in the 'orders' table matches the 'customer_id' column in the 'customer' table,
-- the 'salesman_id' column in the 'orders' table matches the 'salesman_id' column in the 'salesman' table,
-- and ensures that 'salesman.city' and 'customer.grade' are not NULL.
SELECT customer.cust_name AS "Customer", customer.grade AS "Grade", orders.ord_no AS "Order No."
-- Specifies the tables from which to retrieve the data (in this case, 'orders', 'salesman', and 'customer').
FROM orders, salesman, customer
-- Specifies the conditions for joining the tables and filtering the data.
WHERE orders.customer_id = customer.customer_id
AND orders.salesman_id = salesman.salesman_id
AND salesman.city IS NOT NULL
AND customer.grade IS NOT NULL;
Output of the query:
Customer |Grade|Order No| --------------|-----|--------| Nick Rimando | 100| 70002| Geoff Cameron | 100| 70004| Brad Davis | 200| 70005| Nick Rimando | 100| 70008| Fabian Johnson| 300| 70010| Geoff Cameron | 100| 70003| Jozy Altidor | 200| 70011| Nick Rimando | 100| 70013| Graham Zusi | 200| 70001| Graham Zusi | 200| 70007| Julian Green | 300| 70012|
Code Explanation:
The said query in SQL that joins the 'orders', 'salesman', and 'customer' tables. The result set includes the customer name (cust_name) with an alias "Customer", customer grade (grade) with an alias "Grade", and order number (ord_no) with an alias "Order No."
The WHERE clause specifies multiple conditions for the join. A join condition is specified by the first and second conditions between the orders table, and the customer and salesman table, respectively, which states that the customer_id and salesman_id columns of the orders table must match the columns of the customer table and the salesman table, respectively.
The third and fourth conditions specify that the city and grade columns of the salesman and customer tables must not be null, respectively.
Explanation :
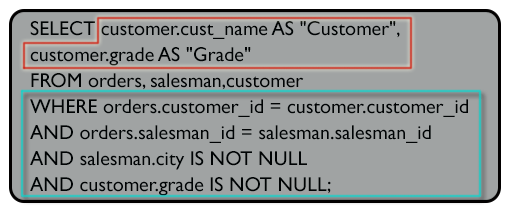
Visual presentation :
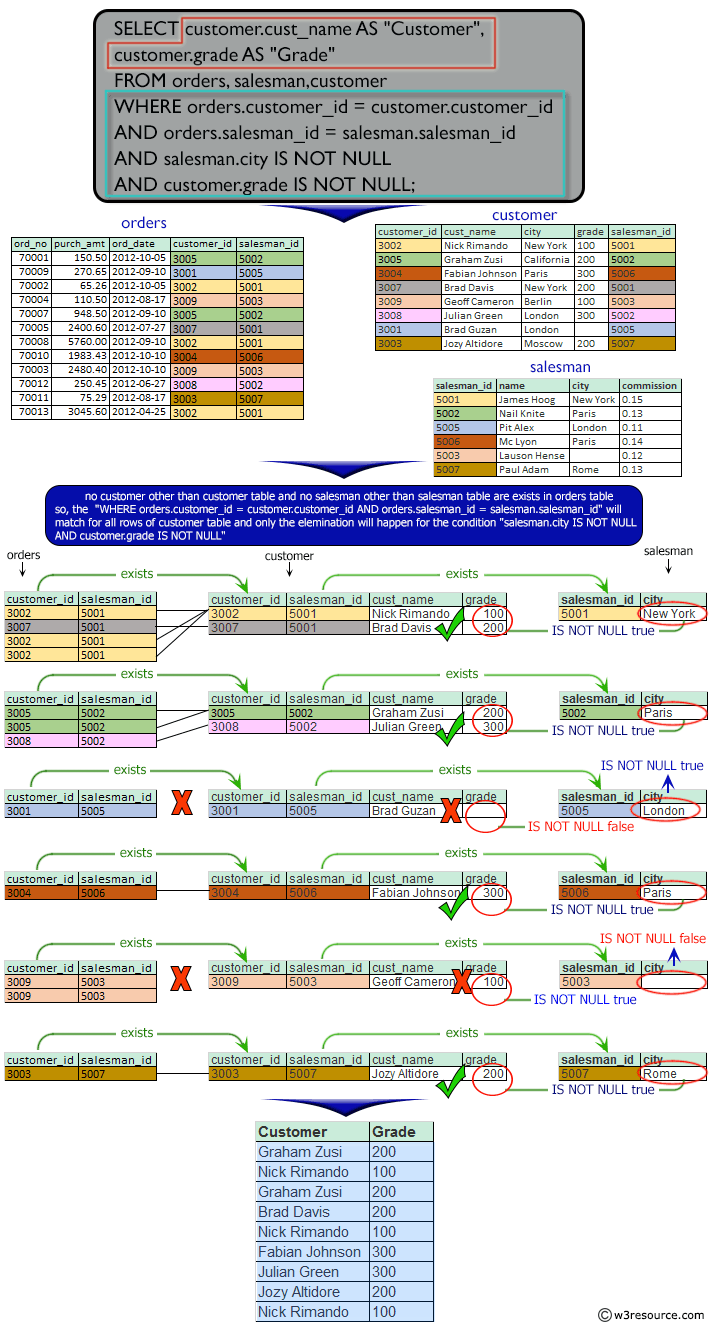
N.B. the column "Order No." has not been shown in the picture.
Practice Online
Query Visualization:
Duration:
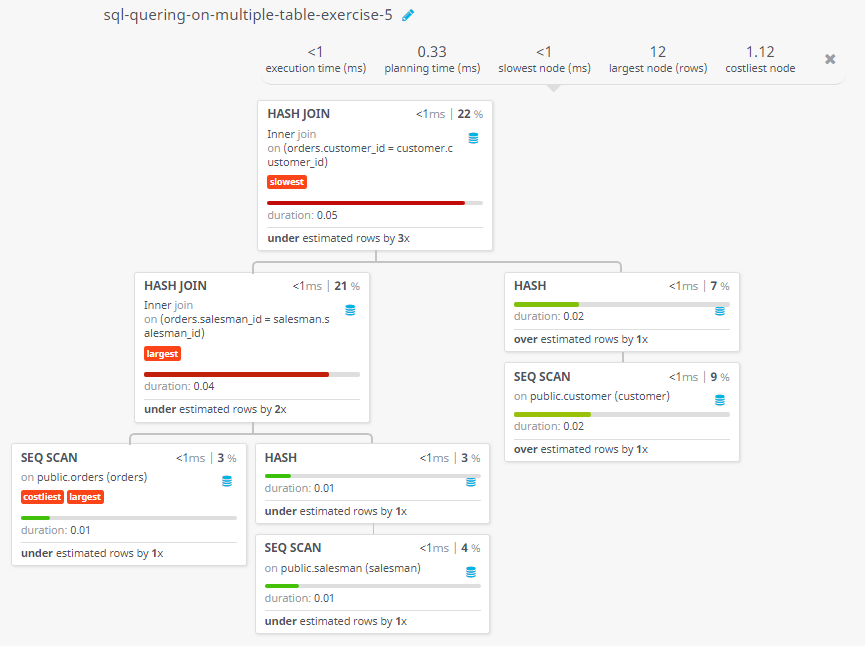
Rows:
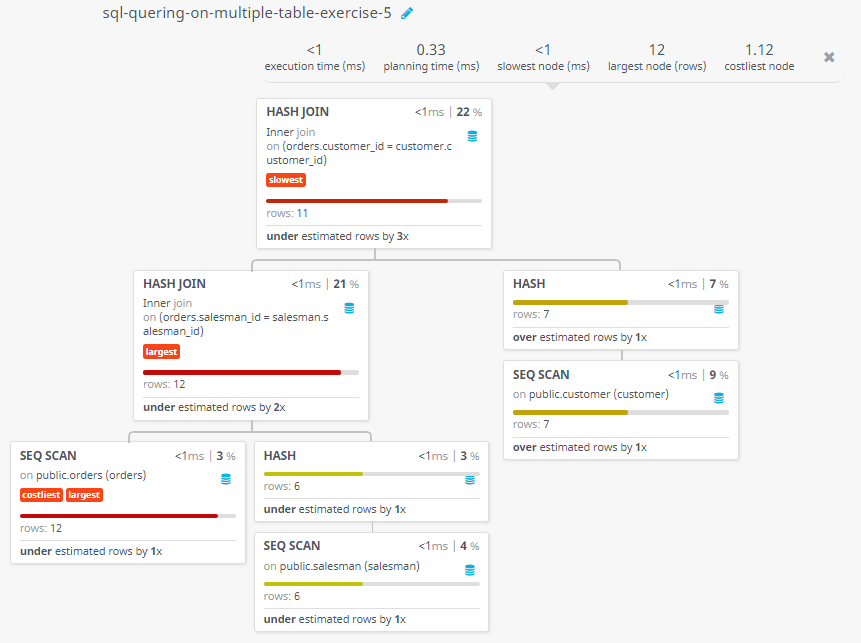
Cost:
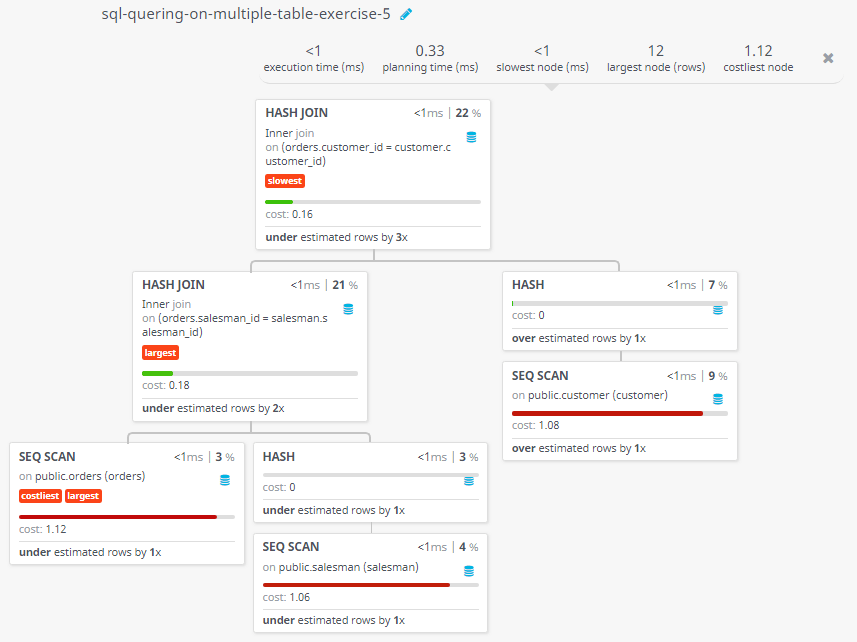
For more Practice: Solve these Related Problems:
- Write a SQL query to list customer names as "Customer" and grades as "Grade" where the customer’s assigned salesperson has a non-null city.
- Write a SQL query to display customers with valid grades and ensure that their salesperson’s city begins with a vowel.
- Write a SQL query to retrieve distinct customer names and grades for customers whose grade is a prime number and whose salesperson’s city is not blank.
- Write a SQL query to list customers as "Customer" and grades as "Grade" for those served by salespeople located in cities with names longer than five characters.
- Write a SQL query to select customers with a grade and exclude those whose salesperson’s city is either 'Unknown' or an empty string.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Find out customers who made the order.
Next SQL Exercise: Customers served by a salesman and commission.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.