SQL Exercises: Largest and smallest orders are produced on each date
4. From the following table, write a SQL query to find the salespersons who generated the largest and smallest orders on each date. Return salesperson ID, name, order no., highest on/lowest on, order date.
Sample table: Salesman
Sample table: Orders
Sample Solution:
-- Selecting specific columns (a.salesman_id, name, ord_no, 'highest on', ord_date) from the 'salesman' table (aliased as 'a') and the 'orders' table (aliased as 'b')
SELECT a.salesman_id, name, ord_no, 'highest on', ord_date
-- Joining the 'salesman' table (aliased as 'a') and the 'orders' table (aliased as 'b') based on the condition that 'salesman_id' is equal in both tables and filtering rows based on the condition that 'purch_amt' is equal to the maximum 'purch_amt' for the same 'ord_date'
FROM salesman a, orders b
WHERE a.salesman_id = b.salesman_id
AND b.purch_amt = (
SELECT MAX(purch_amt)
FROM orders c
WHERE c.ord_date = b.ord_date
)
-- Performing a UNION operation with the result set of a subquery that selects specific columns (a.salesman_id, name, ord_no, 'lowest on', ord_date) from the 'salesman' table (aliased as 'a') and the 'orders' table (aliased as 'b')
UNION
-- Selecting specific columns (a.salesman_id, name, ord_no, 'lowest on', ord_date) from the 'salesman' table (aliased as 'a') and the 'orders' table (aliased as 'b')
SELECT a.salesman_id, name, ord_no, 'lowest on', ord_date
-- Joining the 'salesman' table (aliased as 'a') and the 'orders' table (aliased as 'b') based on the condition that 'salesman_id' is equal in both tables and filtering rows based on the condition that 'purch_amt' is equal to the minimum 'purch_amt' for the same 'ord_date'
FROM salesman a, orders b
WHERE a.salesman_id = b.salesman_id
AND b.purch_amt = (
SELECT MIN(purch_amt)
FROM orders c
WHERE c.ord_date = b.ord_date
)
Sample Output:
salesman_id name ord_no ?column? ord_date 5001 James Hoog 70002 lowest on 2012-10-05 5001 James Hoog 70005 highest on 2012-07-27 5001 James Hoog 70005 lowest on 2012-07-27 5001 James Hoog 70008 highest on 2012-09-10 5001 James Hoog 70013 highest on 2012-04-25 5001 James Hoog 70013 lowest on 2012-04-25 5002 Nail Knite 70001 highest on 2012-10-05 5002 Nail Knite 70012 highest on 2012-06-27 5002 Nail Knite 70012 lowest on 2012-06-27 5003 Lauson Hen 70003 highest on 2012-10-10 5003 Lauson Hen 70004 highest on 2012-08-17 5005 Pit Alex 70009 lowest on 2012-09-10 5006 Mc Lyon 70010 lowest on 2012-10-10 5007 Paul Adam 70011 lowest on 2012-08-17
Code Explanation:
The said query in SQL that selects the salesman_id, name, ord_no, and ord_date for each order where the purchase amount is the highest or lowest for that order date. The query uses a subquery to find the highest or lowest purchase amount for each order date, and then joins the orders and salesman tables using the salesman_id column.
The output of the query will consist of two sets of rows: one for the highest purchase amount and one for the lowest purchase amount for each order date. The 'highest on' and 'lowest on' values are added as literals to indicate which one of the two values it is displaying.
The outer query of the first subquery selects the rows where the purchase amount matches the maximum value.
This UNION keyword combines the results of the first query with the results of the second query.
The outer query of the second subquery selects the rows where the purchase amount matches the minimum value.
Practice Online
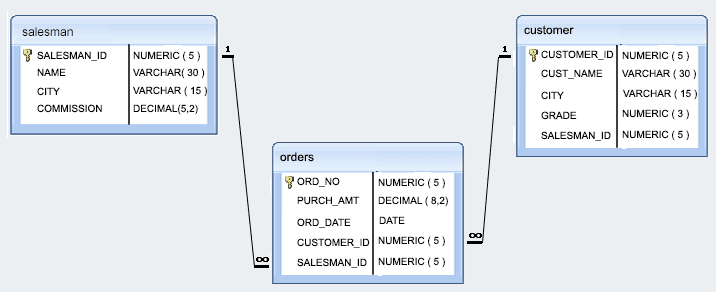
Query Visualization:
Duration:
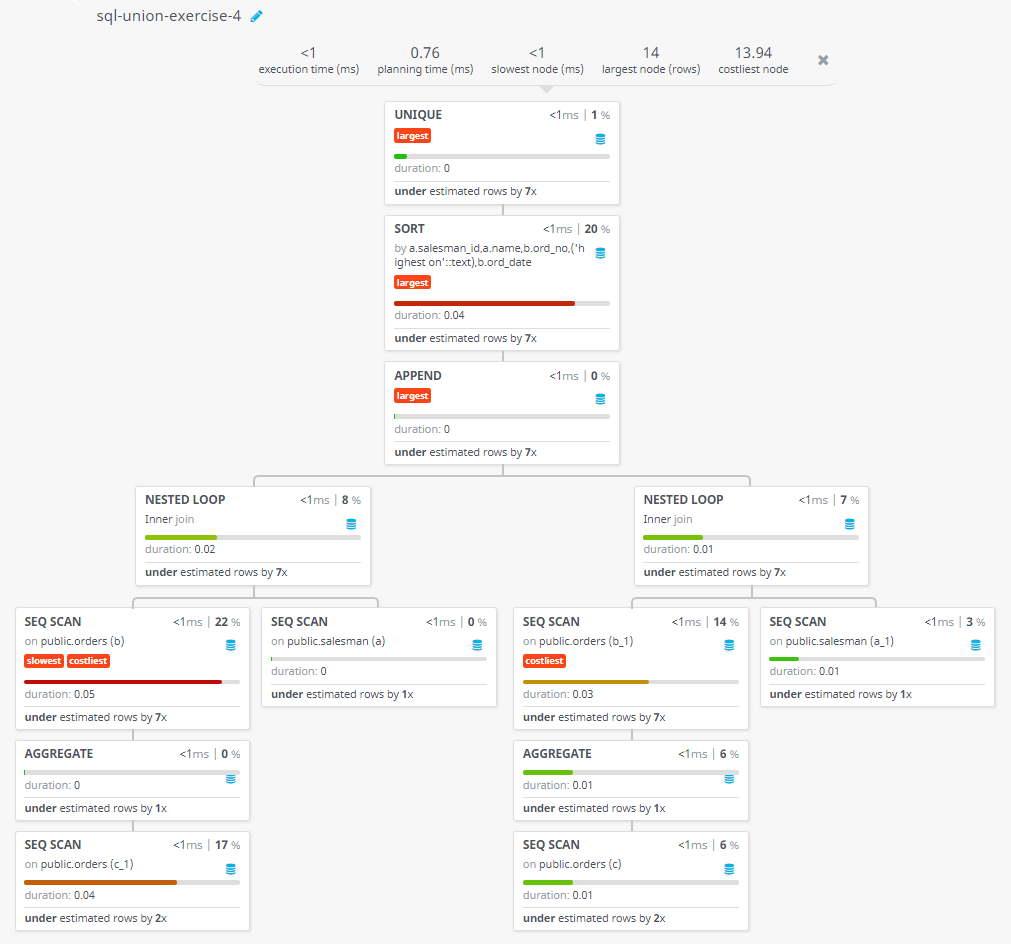
Rows:
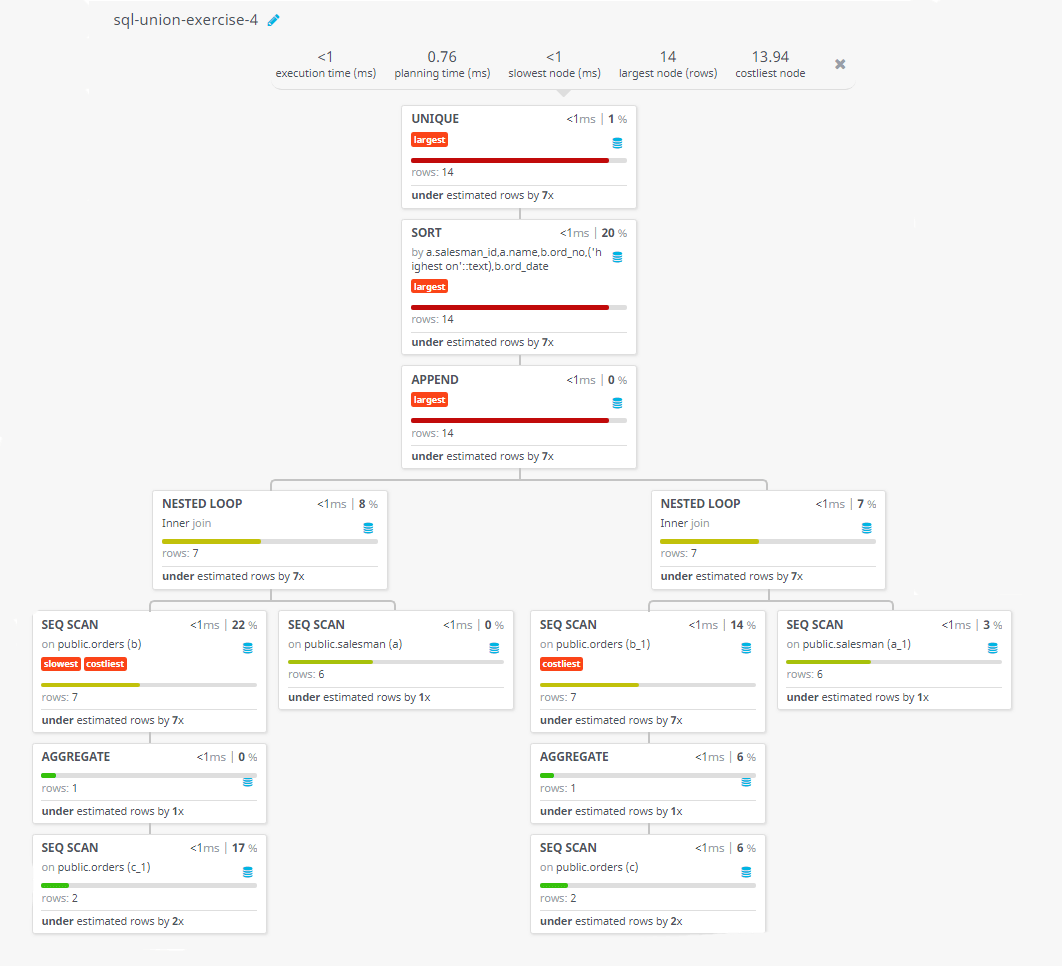
Cost:
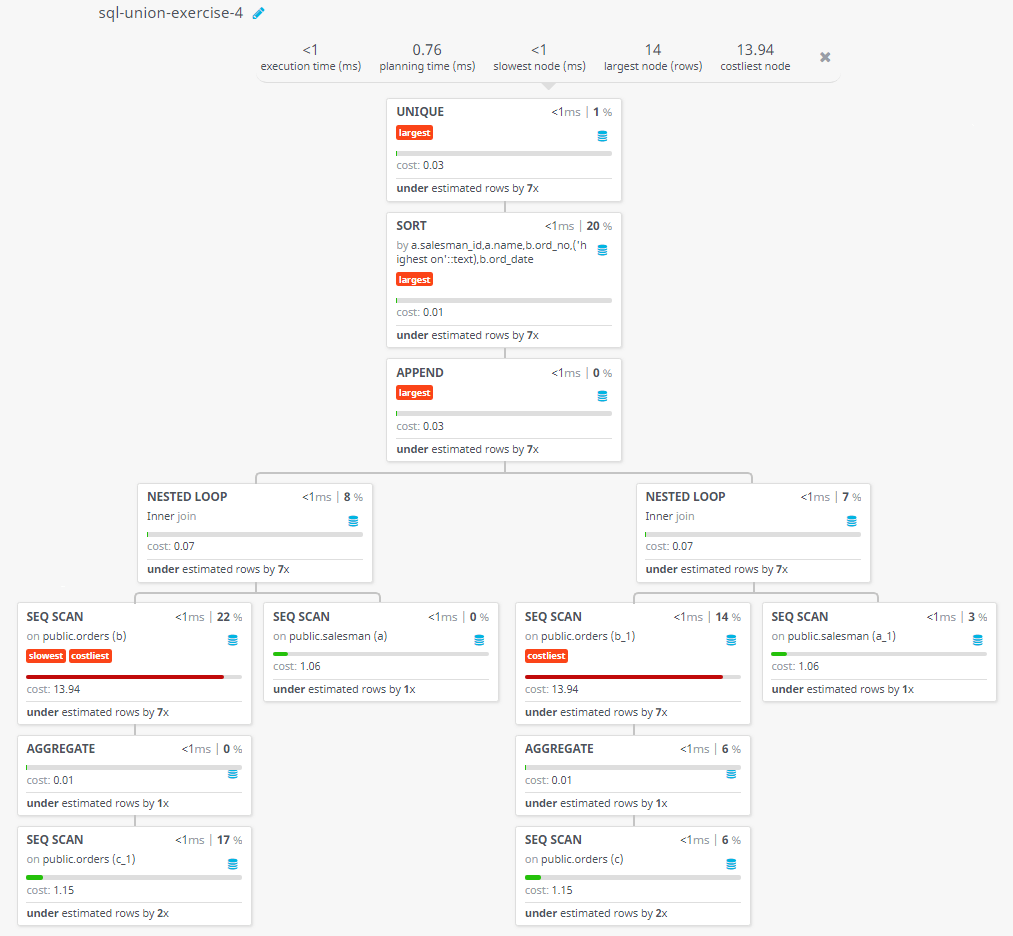
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous SQL Exercise: Salesmen, customer involved in inventory management.
Next SQL Exercise: Largest and smallest orders on each date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.