C Exercises: Check whether a given number is prime or not
32. Prime Number Determination
Write a C program to determine whether a given number is prime or not.
The program should prompt the user for an integer input, then determine if the number has any divisors other than 1 and itself. If the number has no other divisors, it is prime; otherwise, it is not. The result should be displayed to the user.
Visual Presentation:
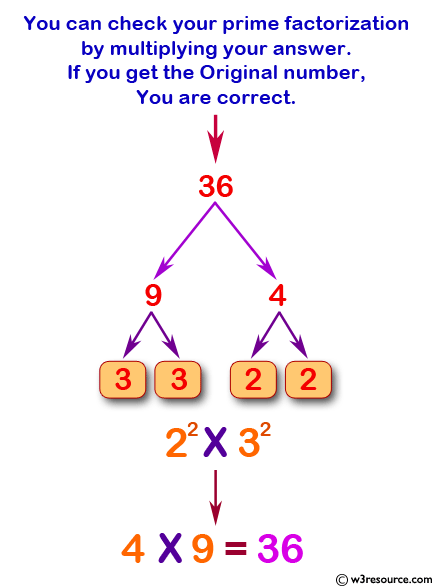
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main(){
int num,i,ctr=0; // Declare variables for user input, loop control, and a counter.
printf("Input a number: "); // Prompt the user to input a number.
scanf("%d",&num); // Read the input number from the user.
for(i=2;i<=num/2;i++){ // Start a loop to check for factors of the input number.
if(num % i==0){ // If the input number is divisible by 'i'.
ctr++; // Increment the counter.
break; // Exit the loop since a factor has been found.
}
}
if(ctr==0 && num!= 1) // If no factors were found and the number is not 1.
printf("%d is a prime number.\n",num); // Print that the number is prime.
else
printf("%d is not a prime number",num); // Print that the number is not prime.
}
Output:
Input a number: 13 13 is a prime number.
Flowchart:
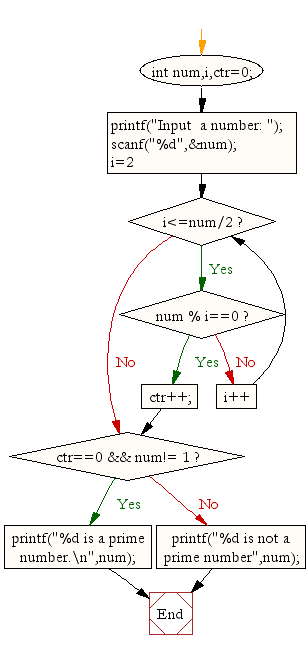
For more Practice: Solve these Related Problems:
- Write a C program to determine if a number is prime using the Euclidean algorithm for optimization.
- Write a C program to check if a number is prime using recursion.
- Write a C program to test primality by checking divisibility only up to the square root of the number.
- Write a C program to check if a number is prime and, if not, display its factors.
C Programming Code Editor:
Previous: Write a program in C to display the pattern like a diamond.
Next: Write a C program to display Pascal's triangle
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.