C Exercises: Display the first n terms of Fibonacci series
35. Fibonacci Series Display
Write a program in C to display the first n terms of the Fibonacci series.
The series is as follows:
Fibonacci series 0 1 2 3 5 8 13 .....
The program should prompt the user for the number of terms, n, and then calculate the Fibonacci sequence up to that number. The Fibonacci series starts with 0 and 1, and each subsequent term is the sum of the previous two terms. The resulting series should be printed to the screen.
Visual Presentation:
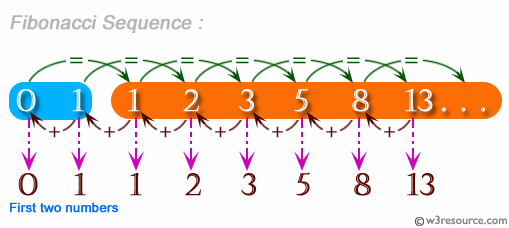
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int prv=0, pre=1, trm, i, n; // Declare variables for previous, current, and next terms, as well as loop counters.
printf("Input number of terms to display : "); // Prompt the user to input the number of terms.
scanf("%d", &n); // Read the input from the user.
printf("Here is the Fibonacci series up to %d terms : \n", n); // Print a message indicating the number of terms.
printf("% 5d % 5d", prv, pre); // Print the first two terms.
for(i=3; i<=n; i++) // Loop to generate the Fibonacci series starting from the third term.
{
trm = prv + pre; // Calculate the next term.
printf("% 5d", trm); // Print the next term.
prv = pre; // Update the previous term.
pre = trm; // Update the current term.
}
printf("\n"); // Move to the next line after printing the series.
}
Output:
Input number of terms to display : 10 Here is the Fibonacci series upto to 10 terms : 0 1 1 2 3 5 8 13 21 34
Flowchart:
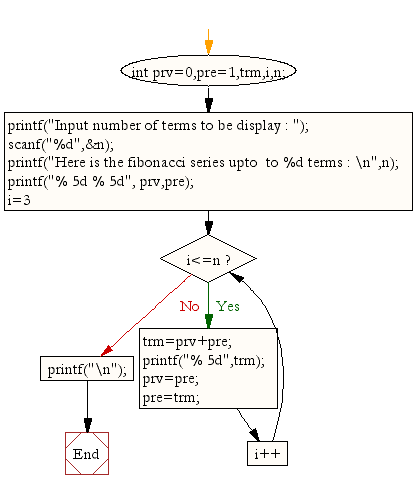
For more Practice: Solve these Related Problems:
- Write a C program to display the first n Fibonacci numbers using recursion.
- Write a C program to print the Fibonacci series and then display the ratio of consecutive terms.
- Write a C program to generate the Fibonacci series using an iterative loop with dynamic memory allocation.
- Write a C program to display the Fibonacci series and compute its total sum.
C Programming Code Editor:
Previous: Write a program in C to find the prime numbers within a range of numbers.
Next: Write a program in C to display the such a pattern for n number of rows using a number which will start with the number 1 and the first and a last number of each row will be 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.