C Exercises: Display the pattern like pyramid using the alphabet
40. Alphabet Pyramid Pattern
Write a C program to display the pyramid pattern using the alphabet.
The pattern is as follows :
A A B A A B C B A A B C D C B A
The pattern consists of rows where each row contains alphabets in a specific sequence. The program should prompt the user for the number of rows, then print the alphabet pyramid accordingly, with each row centered and the alphabets increasing and decreasing symmetrically.
Visual Presentation:
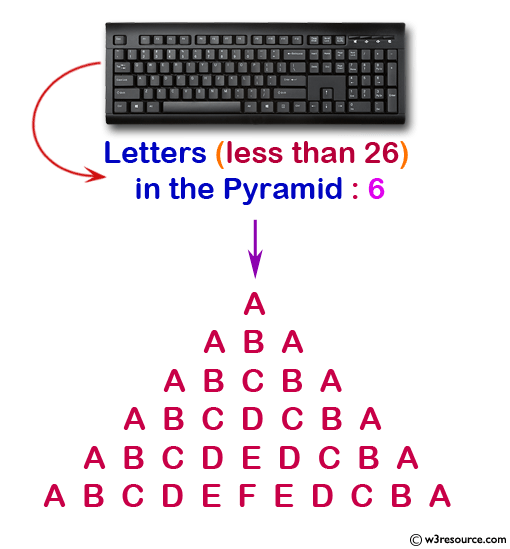
For more Practice: Solve these Related Problems:
- Write a C program to display an alphabet pyramid with letters in ascending order and then in descending order.
- Write a C program to print an alphabet pyramid where each row starts with 'A' and increases sequentially.
- Write a C program to display a centered alphabet pyramid and then convert all letters to lowercase.
- Write a C program to generate an alphabet pyramid and then print the reverse of each row.
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, j; // Declare variables for iteration.
char alph = 'A'; // Initialize a character variable to 'A'.
int n, blk; // Declare variables for user input and block counter.
int ctr = 1; // Initialize a counter.
printf("Input the number of Letters (less than 26) in the Pyramid : ");
scanf("%d", &n); // Prompt user for input and store it in variable 'n'.
for (i = 1; i <= n; i++) // Outer loop for rows.
{
for(blk = 1; blk <= n - i; blk++) // Loop to print spaces before letters.
printf(" ");
for (j = 0; j <= (ctr / 2); j++) // Loop to print letters in ascending order.
{
printf("%c ", alph++);
}
alph = alph - 2; // Decrement the character after printing half.
for (j = 0; j < (ctr / 2); j++) // Loop to print letters in descending order.
{
printf("%c ", alph--);
}
ctr = ctr + 2; // Increment the counter for the next row.
alph = 'A'; // Reset the character to 'A' for the next row.
printf("\n"); // Move to the next line after printing a row.
}
}
Output:
Input the number of Letters (less than 26) in the Pyramid : 6 A A B A A B C B A A B C D C B A A B C D E D C B A A B C D E F E D C B A
Flowchart:
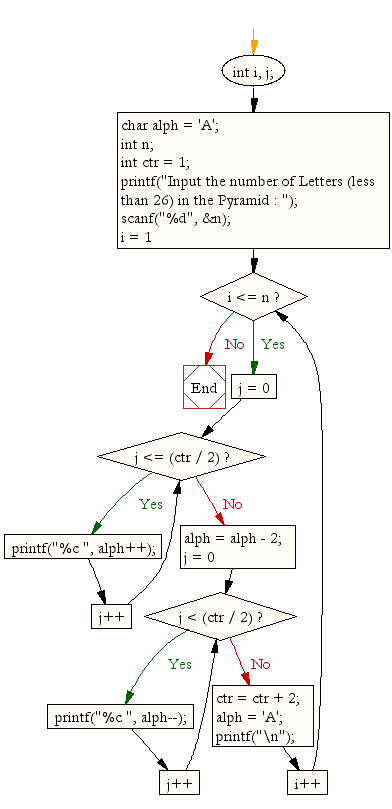
C Programming Code Editor:
Previous: Write a program in C to find the number and sum of all integer between 100 and 200 which are divisible by 9.
Next: Write a program in C to convert a decimal number into binary without using an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.