C Exercises: Convert binary number to octal
53. Binary to Octal Conversion
Write a C program to convert a binary number to octal.
This C program converts a binary number to its equivalent octal representation. It prompts the user to input a binary number, then iterates through each digit to calculate its decimal equivalent. Afterward, it converts the decimal number obtained to octal format, storing and printing both the original binary number and its octal equivalent.
Visual Presentation:
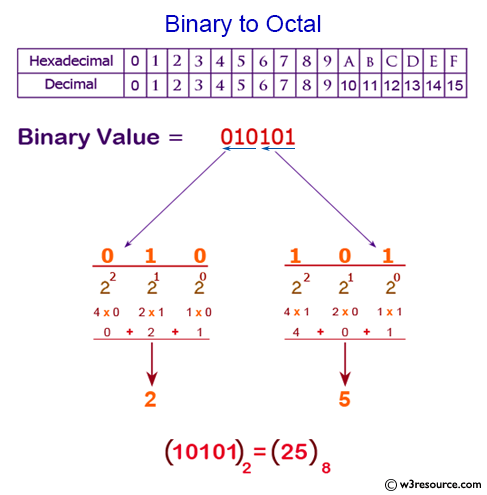
Sample Solution:
C Code:
#include
#include
int main() {
// Declare variables
int n1, n, p = 1;
int dec = 0, i = 0, d;
int ocno = 0, dn;
// Print output headers
printf("\n\nConvert Binary to Octal:\n ");
printf("-------------------------\n");
// Get binary input
printf("Input a binary number: ");
scanf("%d", &n);
// Store input number
n1 = n;
// Convert binary to decimal
// Loop through each binary digit
while (n > 0) {
// Get current digit
d = n % 10;
// Add digit * power of 2 to result
dec += d * pow(2, i);
// Increment power
i++;
// Divide binary number
n = n / 10;
}
// Convert decimal to octal
dn = dec; // Store decimal number
i = 1; // Reset power
// Repeatedly divide decimal by 8
while (dec > 0) {
// Get remainder and add to result
ocno += (dec % 8) * i;
// Update power of 8
i = i * 10;
// Divide decimal number
dec = dec / 8;
}
// Print binary and octal result
printf("\nThe Binary Number: %d\nThe equivalent Octal Number: %d\n\n", n1, ocno);
return 0;
}
Output:
Convert Binary to Octal: ------------------------- Input a binary number :1001 The Binary Number : 1001 The equivalent Octal Number : 11
Explanation
Here's a brief explanation.
- Header and Declarations:
- Includes the necessary header files ('stdio.h' for input/output and 'math.h' for mathematical functions).
- Declare variables 'n1' (input binary number), 'n' (temporary variable for input processing), 'p' (power of 2 multiplier), 'dec' (resulting decimal number), 'd' (temporary variable for digit extraction), 'ocno' (resulting octal number), 'dn' (backup of decimal number), i (loop variable).
- User input:
- Asks the user to input a binary number.
- Reads the input and stores it in the variable 'n'.
- Binary to Decimal Conversion (using a for loop):
- Iterates through each binary number digit using a "for" loop.
- Extracts the current digit and calculates its contribution to the decimal number using the 'pow' function.
- Accumulates the result in the variable 'dec'.
- Convert Decimal to Octal (using a for loop):
- Initialize 'ocno' to 0 and i to 1 for the octal conversion.
- Repeatedly divides the decimal number ('dec') by 8 using a "for" loop.
- Calculates the remainder and adds it to the octal result ('ocno').
- Update the power of 8 (i).
- Continue until the decimal number becomes 0.
- Result Display:
- Prints the input binary number and its equivalent octal number.
- Return Statement:
- Returns 0 to indicate successful execution.
Flowchart
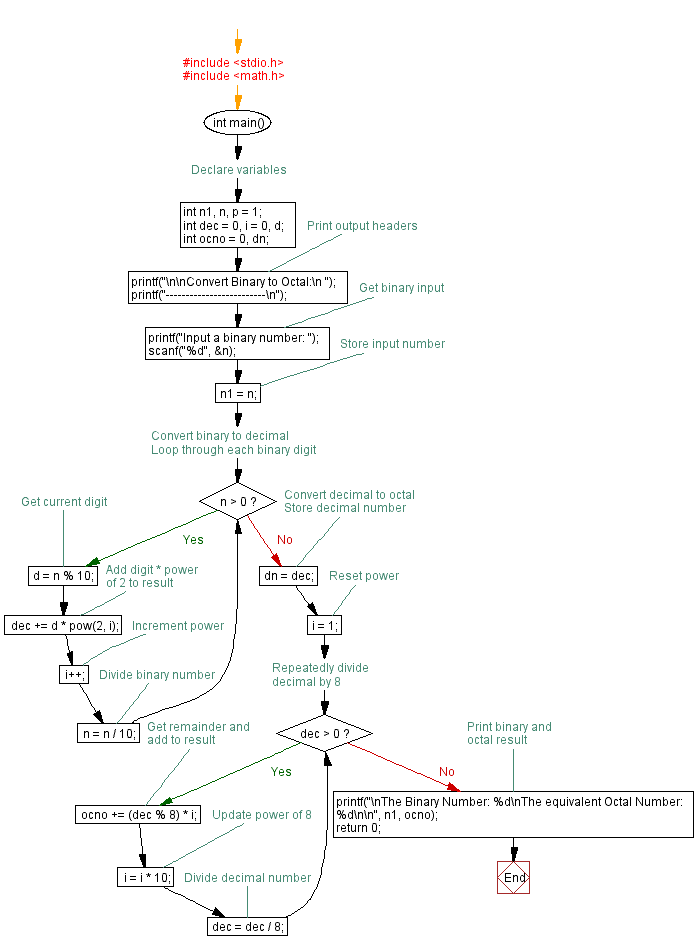
For more Practice: Solve these Related Problems:
- Write a C program to convert a binary number to octal by first converting it to decimal and then to octal.
- Write a C program to convert a binary string to its octal representation using bitwise operations.
- Write a C program to convert a binary number to octal without using arrays by processing bits in groups of three.
- Write a C program to convert a binary number to octal and verify the result by converting it back to binary.
C Programming Code Editor:
Previous: Write a program in c to find the Sum of GP series.
Next: Write a program in C to convert an octal number into binary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.