C Exercises: Find the length of a string without using the library function
58. Find String Length Without Library Function
Write a C program to find the length of a string without using the library function.
This C program takes a string as input and iterates through each character until it encounters the null terminator '\0', incrementing a counter variable for each character. After iterating through the entire string, it prints the count of characters as the length of the string.
Visual Presentation:
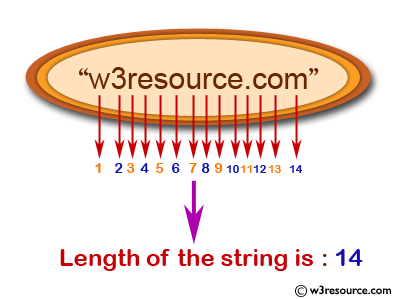
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <string.h>
void main()
{
// Variable declarations
char str1[50];
int i, l = 0;
// Prompting user for input
printf("\n\nFind the length of a string:\n ");
printf("-------------------------------------\n");
// Getting user input
printf("Input a string : ");
scanf("%s", str1);
// Loop to count characters in the string
for (i = 0; str1[i] != '\0'; i++)
{
l++;
}
// Printing the length of the string
printf("The string contains %d number of characters. \n", l);
printf("So, the length of the string %s is : %d\n\n", str1, l);
}
Output:
Find the length of a string: ------------------------------------- Input a string : welcome The string contains 7 number of characters. So, the length of the string welcome is : 7
Flowchart:
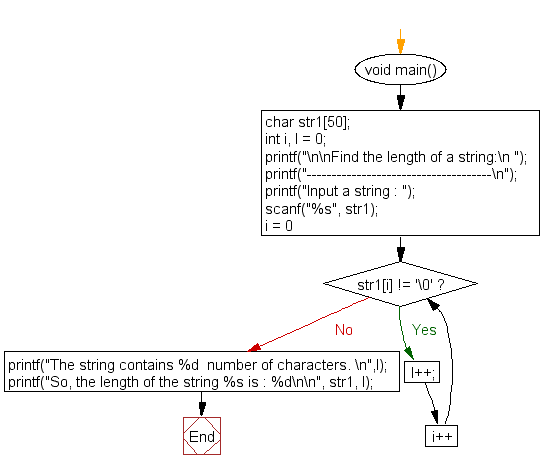
For more Practice: Solve these Related Problems:
- Write a C program to calculate the length of a string using pointer arithmetic without using strlen().
- Write a C program to find the length of a string recursively without any loop constructs.
- Write a C program to compute the length of a string by traversing each character without standard library functions.
- Write a C program to determine the length of a string and then compare it with a user-provided value.
C Programming Code Editor:
Previous: Write a program in C to print a string in reverse order.
Next: Write a program in C to check Armstrong number of n digits.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.