HTML5 Canvas: Gradients and Patterns
Introduction
In HTML5 canvas, you can fill or stroke shapes and text using stroke() or fill() method. When we draw on text or shapes it uses the current stroke or fill style. The stroke or fill style can be set to a color, a pattern, or a gradient.
Gradients
Gradients consist of continuously smooth color transitions along a vector from one color to another, possibly followed by additional transitions along the same vector to other colors. HTML5 canvas (2D) supports two kinds of gradient : linear and radial.
Linear gradient:
A linear gradient defines color change along a line between two points. You can create a linear gradient by calling createLinearGradient() method. Here is the syntax and parameters of the method:
Syntax:
var gradient = ctx.createLinearGradient(x0, y0, x1, y1);
Parameters | Type | Description |
---|---|---|
x0 |
number | The x-coordinate (in pixels), of the start point of the gradient. |
y0 | number | The y-coordinate (in pixels), of the start point of the gradient. |
x1 | number | The x-coordinate (in pixels), of the end point of the gradient. |
y1 | number | The y-coordinate (in pixels), of the end point of the gradient. |
The next step in defining a gradient is to add at least two color stops. To do this use addColorStop() method. Here is the syntax and parameters of the method :
Syntax:
gradient.addColorStop(offset, color);
Parameters | Type | Description |
---|---|---|
offset | number | A floating point value between 0.0 and 1.0 that represents the position where 0 is the beginning of the gradient and 1 is the end.. |
color | number | A CSS color string to display at the position that the offset parameter specifies. |
Example: HTML5 Canvas Linear gradient
The following web document creates a gradient:
Output:
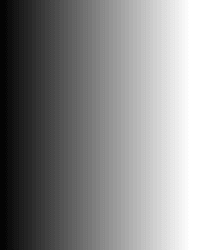
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Linear gradient example</title>
</head>
<body>
<canvas id="demoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("demoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext("2d");
var gradient = ctx.createLinearGradient(10, 90, 200, 90);
gradient.addColorStop(0, 'black');
gradient.addColorStop(1, 'white');
ctx.fillStyle = gradient;
ctx.fillRect(10, 10, 200, 250);
}
</script>
</body>
</html>
Example: HTML5 Canvas Linear gradient using RGBa() color value
You can create a gradient by specifying a color stop with an RGBa() color value which includes degrees of transparency. The following example draws four rectangles, each with a gradient going in a different direction, all going from red to 50% transparent blue.
Output :
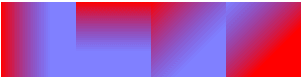
Code:
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Linear gradinet example</title>
</head>
<body>
<canvas id="demoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("demoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext("2d");
var gradient1 = ctx.createLinearGradient(0, 0, 50, 0);
gradient1.addColorStop(0, 'red');
gradient1.addColorStop(1, 'rgba(0, 0, 255, 0.5)');
ctx.fillStyle = gradient1;
ctx.fillRect(0, 0, 75, 75);
var gradient2 = ctx.createLinearGradient(0, 0, 0, 50);
gradient2.addColorStop(0, 'red');
gradient2.addColorStop(1, 'rgba(0, 0, 255, 0.5)');
ctx.fillStyle = gradient2;
ctx.fillRect(75, 0, 75, 75);
var gradient3 = ctx.createLinearGradient(150, 0, 200, 50);
gradient3.addColorStop(0, 'red');
gradient3.addColorStop(1, 'rgba(0, 0, 255, 0.5)');
ctx.fillStyle = gradient3;
ctx.fillRect(150, 0, 75, 75);
var gradient3 = ctx.createLinearGradient(275, 50, 225, 0);
gradient3.addColorStop(0, 'red');
gradient3.addColorStop(1, 'rgba(0, 0, 255, 0.5)');
ctx.fillStyle = gradient3;
ctx.fillRect(225, 0, 75, 75);
}
</script>
</body>
</html>
Example: HTML5 Canvas Rainbow gradient
The following example creates a rainbow gradient with seven color, each color has 1/6th of the distance along the gradient.
Output :

Code:
<!DOCTYPE html>
<html>
<head>
<title>HTML5 Canvas - Rainbow Gradient</title>
</head>
<body>
<canvas id="demoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("demoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext("2d");
var gradient = ctx.createLinearGradient(10, 0, 500, 0);
gradient.addColorStop(0, 'red');
gradient.addColorStop(1 / 6, 'orange');
gradient.addColorStop(2 / 6, 'yellow');
gradient.addColorStop(3 / 6, 'green');
gradient.addColorStop(4 / 6, 'blue');
gradient.addColorStop(5 / 6, 'indigo');
gradient.addColorStop(1, 'violet');
ctx.fillStyle = gradient;
ctx.fillRect(0, 0, 500, 75);
}
</script>
</body>
</html>
Radial gradient:
A radial gradient defines a color change along a cone (a three-dimensional geometric shape) between two circles. You can create a radial gradient by calling createRadialGradient() method and passing in two circles and also providing source and destination circle radius.
Syntax:
var gradient = ctx.createRadialGradient(x0, y0, r0, x1, y1, r1)
Parameters | Type | Description |
---|---|---|
x0 | number | The x-coordinate (in pixels), of the starting circle of the gradient. |
y0 | number | The y-coordinate (in pixels), of the starting circle of the gradient. |
rad0 | number | The radius of the starting circle. |
x1 | number | The x-coordinate (in pixels), of the ending circle of the gradient. |
y1 | number | The y-coordinate (in pixels), of the ending circle of the gradient. |
rad1 | number | The radius of the ending circle. |
The next step is to add at least two color stops. To do this use addColorStop() method.
Example -1 : HTML5 Canvas Radial gradient
The following web document defines a radial gradient and uses it to fill a circle.
Output:
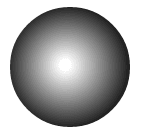
Code :
<!DOCTYPE html>
<html>
<head>
<title>Example : HTML5 Canvas Radial gradient example1 </title>
</head>
<body>
<canvas id="demoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("demoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext("2d");
var gradient = ctx.createRadialGradient(85, 88, 5, 88, 90, 69);
gradient.addColorStop(0, 'white');
gradient.addColorStop(1, 'black');
ctx.fillStyle = gradient;
ctx.arc(90, 90, 60, 0, 2 * Math.PI);
ctx.fill();
}
</script>
</body>
</html>
Example -2 : HTML5 Canvas Radial gradient
In the following web document we have defined two different gradients. As we can control the start and closing points of the gradient, we can create more complex effects. Here, we've offset the starting point slightly from the end point to achieve a spherical 3D effect.
Output:
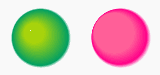
Code:
<!DOCTYPE html>
<html>
<head>
<title>Example : HTML5 Canvas Radial gradient example2 </title>
</head>
<body>
<canvas id="demoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("demoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext("2d");
// Create gradients
var g1 = ctx.createRadialGradient(45,45,10,52,50,30);
g1.addColorStop(0, '#A7D30C');
g1.addColorStop(0.9, '#019F62');
g1.addColorStop(1, 'rgba(1,159,98,0)');
var g2 = ctx.createRadialGradient(125,45,20,132,50,30);
g2.addColorStop(0, '#FF5F98');
g2.addColorStop(0.75, '#FF0188');
g2.addColorStop(1, 'rgba(255,1,136,0)');
// draw shapes
ctx.fillStyle = g1;
ctx.fillRect(0,0,150,150);
ctx.fillStyle = g2;
ctx.fillRect(100,0,170,150);
}
</script>
</body>
</html>
Patterns
A pattern is used to fill or stroke an object using a pre-defined graphic object which can be replicated ("tiled") at fixed intervals in x and y to cover the areas to be painted. A pattern consists of an image source—such as a JPG, GIF, PNG, SVG, or the currently playing frame of a video—and a repetition setting, to create a pattern from tiles of the image.
You create a pattern using the context’s createPattern() method, passing in the image object and a "repeat" parameter.
Syntax:
var pattern = ctx.createPattern(image, repetition);
Parameters | Type | Description |
---|---|---|
image | Object | An image, canvas, or video element of the pattern to use. |
repetition | number | The direction of repetition. The "repeat" string repeats the image both horizontally and vertically, but you can specify one of the following values- repeat : The pattern repeats in both horizontal and vertical directions. repeat-x : The pattern repeats only in the horizontal direction. repeat-y : The pattern repeats only in the vertical direction. no-repeat : The pattern prints only once and does not repeat. |
Example -1 : HTML5 Canvas patterns
The following example loads two images - pattern1.png and pattern2.png and makes them into patterns that are each used to stroke a line and fill a rectangle.
Output:
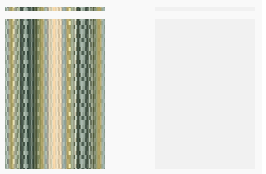
Code :
<!DOCTYPE html>
<html>
<head>
<title>Example : HTML5 Canvas Pattern example </title>
</head>
<body>
<canvas id="demoCanvas" width="500" height="600"></canvas>
<script>
var canvas = document.getElementById("demoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext("2d");
// create new image object to use as pattern
var image1 = new Image();
var image2 = new Image();
image1.src = 'http://www.w3resource.com/html5-canvas/images/pattern1.png';
image2.src = 'http://www.w3resource.com/html5-canvas/images/pattern3.png';
var pat1 = ctx.createPattern(image1, "repeat");
var pat2 = ctx.createPattern(image2, "repeat");
ctx.lineWidth = 4;
// stroke line and fill rect with block pattern
ctx.beginPath();
ctx.moveTo(50, 10);
ctx.lineTo(150, 10);
ctx.strokeStyle = pat1;
ctx.stroke();
ctx.fillStyle = pat1;
ctx.fillRect(50, 20, 100, 150);
// stroke line and fill rect with cork pattern
ctx.beginPath();
ctx.moveTo(200, 10);
ctx.lineTo(300, 10);
ctx.strokeStyle = pat2;
ctx.stroke();
ctx.fillStyle = pat2;
ctx.fillRect(200, 20, 100, 150);
}
</script>
</body>
</html>
Code Editor:
See the Pen html css common editor by w3resource (@w3resource) on CodePen.
Previous: HTML5 Canvas Rectangle
Next:
HTML5 Canvas Text