JavaScript: Check whether two numbers are in range 40..60 or in the range 70..100 inclusive
JavaScript Basic: Exercise-33 with Solution
Check if Two Numbers are in Specific Ranges
Write a JavaScript program to check whether two numbers are in the range 40..60 or 70..100 inclusive.
This JavaScript program checks if two given numbers fall within either the range 40 to 60 or the range 70 to 100, inclusive. It returns true if both numbers fall within any of these ranges, and false otherwise.
Visual Presentation:
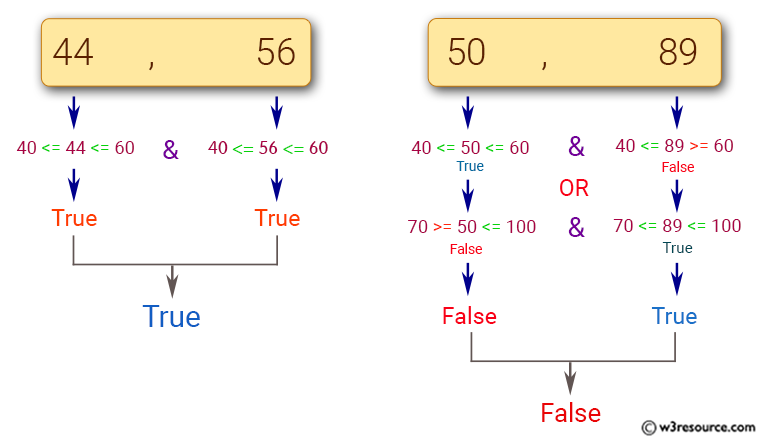
Sample Solution:
JavaScript Code:
// Define a function named numbers_ranges with parameters x and y
function numbers_ranges(x, y) {
// Check if x and y fall within the first range (40 to 60) or the second range (70 to 100)
if ((x >= 40 && x <= 60 && y >= 40 && y <= 60) || (x >= 70 && x <= 100 && y >= 70 && y <= 100)) {
// Return true if the conditions are met
return true;
} else {
// Return false if the conditions are not met
return false;
}
}
// Log the result of calling numbers_ranges with the arguments 44 and 56 to the console
console.log(numbers_ranges(44, 56));
// Log the result of calling numbers_ranges with the arguments 70 and 95 to the console
console.log(numbers_ranges(70, 95));
// Log the result of calling numbers_ranges with the arguments 50 and 89 to the console
console.log(numbers_ranges(50, 89));
Output:
true true false
Live Demo:
See the Pen JavaScript: check if two numbers are in a range - basic- ex-33 by w3resource (@w3resource) on CodePen.
Flowchart:
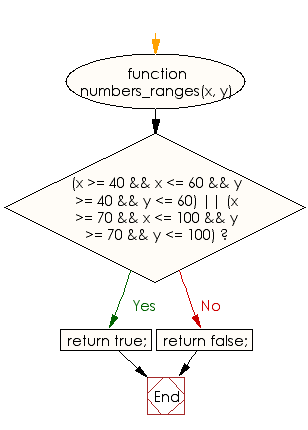
ES6 Version:
// Define a function named numbers_ranges using arrow function syntax with parameters x and y
const numbers_ranges = (x, y) => {
// Check if x and y fall within the specified ranges using the logical OR operator
return (
(x >= 40 && x <= 60 && y >= 40 && y <= 60) ||
(x >= 70 && x <= 100 && y >= 70 && y <= 100)
);
};
// Log the result of calling numbers_ranges with the arguments 44 and 56 to the console
console.log(numbers_ranges(44, 56));
// Log the result of calling numbers_ranges with the arguments 70 and 95 to the console
console.log(numbers_ranges(70, 95));
// Log the result of calling numbers_ranges with the arguments 50 and 89 to the console
console.log(numbers_ranges(50, 89));
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if two numbers fall into either the range 40..60 or the range 70..100, returning true if both conditions are met.
- Write a JavaScript program that validates two numbers to determine if one falls in the 40..60 range and the other in the 70..100 range.
- Write a JavaScript program that accepts two numbers and reports which specific range (40-60 or 70-100) each number belongs to.
Go to:
PREV : Find Closest Value to 100 from Two Numbers.
NEXT : Find Larger Number in Range 40–60.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.