JavaScript: Count the number of days passed since beginning of the year
JavaScript Datetime: Exercise-16 with Solution
Days Passed in Year
Write a JavaScript function to count the number of days passed since the year began.
Test Data:
console.log(days_passed(new Date(2015, 0, 15)));
15
console.log(days_passed(new Date(2015, 11, 14)));
348
Sample Solution:
JavaScript Code:
// Define a JavaScript function called days_passed with parameter dt
function days_passed(dt) {
// Create a copy of the provided date using getTime() method to avoid modifying the original date
var current = new Date(dt.getTime());
// Create a new Date object representing January 1st of the same year as the provided date
var previous = new Date(dt.getFullYear(), 0, 1);
// Calculate the difference in milliseconds between the provided date and January 1st
// Add 1 to consider the current date itself
// Divide by the number of milliseconds in a day (86400000) to convert milliseconds to days
// Round up to the nearest whole number using Math.ceil()
return Math.ceil((current - previous + 1) / 86400000);
}
// Output the number of days passed since January 1st, 2015
console.log(days_passed(new Date(2015, 0, 15)));
// Output the number of days passed since January 1st, 2015 for a different date
console.log(days_passed(new Date(2015, 11, 14)));
Output:
15 348
Explanation:
In the exercise above,
- The code defines a JavaScript function named "days_passed()" with one parameter 'dt', representing a Date object.
- Inside the function:
- It creates a copy of the provided date (dt) using "getTime()" method to avoid modifying the original date, and stores it in the variable 'current'.
- It creates a new Date object named 'previous', representing January 1st of the same year as the provided date (dt).
- It calculates the difference in milliseconds between the provided date and January 1st of the same year, then adds 1 to consider the current date itself.
- It divides the total milliseconds by the number of milliseconds in a day (86400000) to convert milliseconds to days.
- It rounds up the result to the nearest whole number using "Math.ceil()" to get the number of days passed since January 1st of the same year.
- Finally, it returns the calculated number of days passed.
- The code then demonstrates the usage of the "days_passed()" function by calling it with two different dates:
- January 15th, 2015 (new Date(2015, 0, 15))
- December 14th, 2015 (new Date(2015, 11, 14))
- Each console.log() call outputs the number of days passed since January 1st of the respective year to the console.
Flowchart:
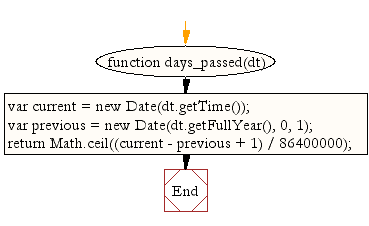
Live Demo:
See the Pen JavaScript - Count the number of days passed since beginning of the year-date-ex- 16 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that calculates how many days have passed since January 1st of the current year using the Date object.
- Write a JavaScript function that subtracts January 1st’s timestamp from the current date’s timestamp and divides by the number of milliseconds per day.
- Write a JavaScript function that accounts for leap years when computing the day of the year for a given Date object.
- Write a JavaScript function that validates the input date and returns the day count as an integer.
Go to:
PREV : Quarter of Year.
NEXT : Unix to Time.
Improve this sample solution and post your code through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.