JavaScript: Get a full numeric representation of a year
JavaScript Datetime: Exercise-28 with Solution
Full Year
Write a JavaScript function to get a full numeric representation of a year (4 digits).
Test Data :
dt = new Date(2015, 10, 1);
console.log(full_year(dt));
2015
Sample Solution:
JavaScript Code:
// Define a JavaScript function called full_year with parameter dt (date)
function full_year(dt)
{
// Return the full year value of the provided date
return dt.getFullYear();
}
// Create a new Date object representing the current date
dt = new Date();
// Output the full year value for the current date
console.log(full_year(dt));
// Create a new Date object representing November 1, 2015
dt = new Date(2015, 10, 1);
// Output the full year value for November 1, 2015
console.log(full_year(dt));
Output:
2018 2015
Explanation:
In the exercise above,
- The code defines a JavaScript function named "full_year()" with one parameter 'dt', representing a Date object.
- Inside the full_year function:
- It retrieves the full year value from the provided Date object "dt" using the "getFullYear()" method.
- It returns the retrieved full year value.
- The code then demonstrates the usage of the "full_year()" function:
- It creates a new Date object "dt" representing the current date using 'new Date()'.
- It outputs the full year value for the current date by calling the "full_year()" function with 'dt' and logging the result to the console.
- It creates another new Date object "dt" representing November 1, 2015, using 'new Date(2015, 10, 1)'.
- It outputs the full year value for November 1, 2015, by calling the "full_year()" function with 'dt' and logging the result to the console.
Flowchart:
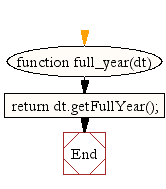
Live Demo:
See the Pen JavaScript - Get a full numeric representation of a year-date-ex-28 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that extracts and returns the full 4-digit year from a Date object.
- Write a JavaScript function that accepts a date string and outputs the full year as a number.
- Write a JavaScript function that validates a Date object and returns the year, handling two-digit inputs appropriately.
- Write a JavaScript function that converts a Date’s year to a string and ensures it is always four digits.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function to get a short textual representation of a month, three letters (Jan through Dec).
Next: Write a JavaScript function to get a two digit representation of a year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.