JavaScript: Reverse a number
JavaScript Function: Exercise-1 with Solution
Reverse Number
Write a JavaScript function that reverses a number.
Sample Data and output:
Example x = 32243;
Expected Output: 34223
Pictorial Presentation:
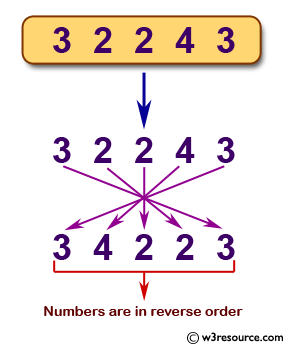
Sample Solution-1:
JavaScript Code:
// Define a function named reverse_a_number that takes a parameter n
function reverse_a_number(n)
{
// Convert the input number to a string
n = n + "";
// Split the string into an array of characters, reverse the array, and then join the characters back into a string
return n.split("").reverse().join("");
}
// Convert the reversed string back to a number and log it to the console
console.log(Number(reverse_a_number(32243)));
Output:
34223
Explanation:
Assume n = 1000.
Convert a number to a string :
Code :
-> n = n + "";
Note : There are different ways to convert number to string :
- String literal -> str = "" + num + "";
- String constructor -> str = String(num);
- toString -> str = num.toString();
- String Literal simple -> str = "" + num;
The split() method is used to split a String object into an array of strings by separating the string into substrings.
Code :
console.log('1000'.split(""));
Output : ["1", "0", "0", "0"]
The reverse() method is used to reverse an array in place. The first array element becomes the last and the last becomes the first.
Code : console.log(["1", "0", "0", "0"].reverse());
Output : ["0", "0", "0", "1"]
The join() method is used to join all elements of an array into a string.
Code : console.log(["1", "0", "0", "0"].reverse().join(""));
The Number constructor contains constants and methods for working with numbers. Values of other types can be converted to numbers using the Number() function.
Output : 1
Flowchart:
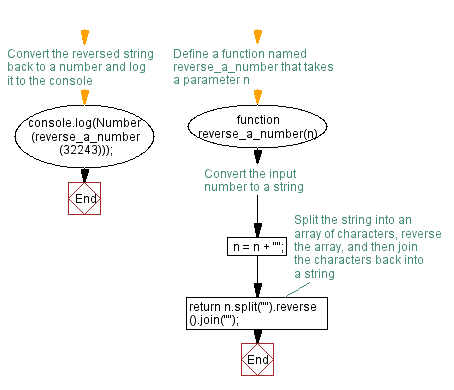
Live Demo:
See the Pen JavaScript -Reverse a number-function-ex- 1 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Define a function named reverse_a_number that takes a parameter num
function reverse_a_number(num) {
// Initialize a variable to store the reversed number
let reversed_num = 0;
// Continue the loop until the input number becomes 0
while (num !== 0) {
// Multiply the current reversed number by 10 and add the last digit of the input number
reversed_num = reversed_num * 10 + num % 10;
// Remove the last digit from the input number
num = Math.floor(num / 10);
}
// Return the reversed number
return reversed_num;
}
// Declare a constant variable num with the value 12345
const num = 12345;
// Log the original number to the console
console.log("Original number: " + num);
// Call the reverse_a_number function with num as an argument and store the result in the variable result
const result = reverse_a_number(num);
// Log the reversed number to the console
console.log("Reversed number: " + result);
Output:
Original number: 12345 Reversed number: 54321
Explanation:
The above function takes a number as input and uses a while loop to reverse the digits of the number. The reversed_num variable is used to store the reversed number, which is initially set to 0. The while loop continues until num is 0, at which point the reversed number is returned.
Flowchart:
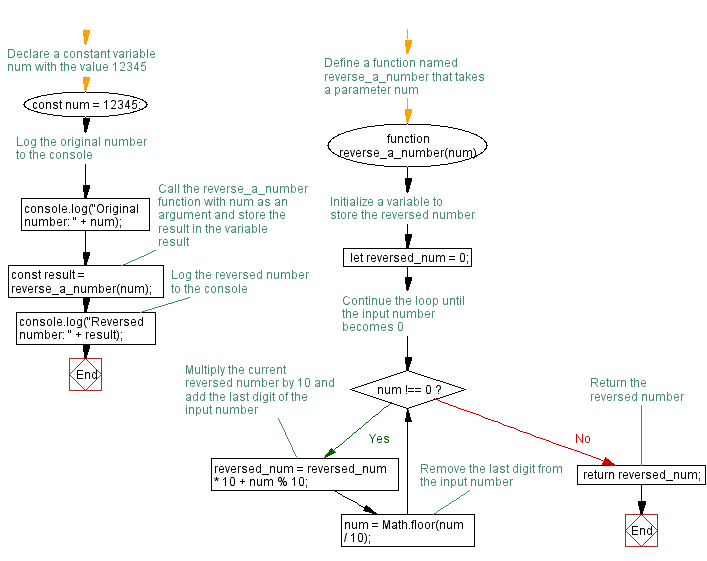
Live Demo:
See the Pen javascript-function-exercise-1-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that reverses the digits of a negative number while preserving its negative sign.
- Write a JavaScript function that reverses the digits of a floating-point number and correctly places the decimal point in the output.
- Write a JavaScript function that reverses a number provided as a string input and handles any leading zeros appropriately.
- Write a JavaScript function that reverses a number using only mathematical operations without converting it to a string.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript program to find the smallest prime number strictly greater than a given number.
Next: Write a JavaScript function that checks whether a passed string is palindrome or not?
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.