JavaScript: Check whether a passed string is palindrome or not
JavaScript Function: Exercise-2 with Solution
Check Palindrome
Write a JavaScript function that checks whether a passed string is a palindrome or not?
Note: A palindrome is word, phrase, or sequence that reads the same backward as forward, e.g., madam or nurses run.
Visual Presentation:
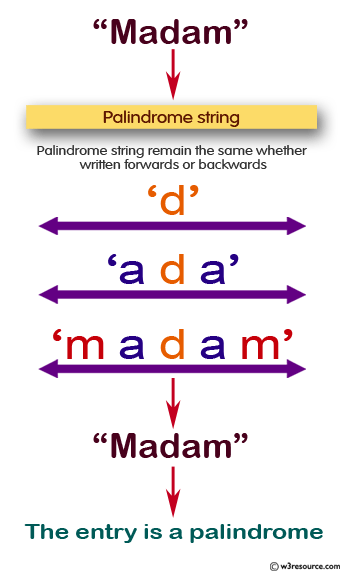
Sample Solution-1:
JavaScript Code:
// Write a JavaScript function that checks whether a passed string is palindrome or not?
function check_Palindrome(str_entry){
// Change the string into lower case and remove all non-alphanumeric characters
var cstr = str_entry.toLowerCase().replace(/[^a-zA-Z0-9]+/g,'');
var ccount = 0;
// Check whether the string is empty or not
if(cstr==="") {
console.log("Nothing found!");
return false;
}
// Check if the length of the string is even or odd
if ((cstr.length) % 2 === 0) {
ccount = (cstr.length) / 2;
} else {
// If the length of the string is 1 then it becomes a palindrome
if (cstr.length === 1) {
console.log("Entry is a palindrome.");
return true;
} else {
// If the length of the string is odd ignore middle character
ccount = (cstr.length - 1) / 2;
}
}
// Loop through to check the first character to the last character and then move next
for (var x = 0; x < ccount; x++) {
// Compare characters and drop them if they do not match
if (cstr[x] != cstr.slice(-1-x)[0]) {
console.log("Entry is not a palindrome.");
return false;
}
}
console.log("The entry is a palindrome.");
return true;
}
check_Palindrome('madam');
check_Palindrome('nursesrun');
check_Palindrome('fox');
Output:
The entry is a palindrome. The entry is a palindrome. Entry is not a palindrome.
Flowchart:
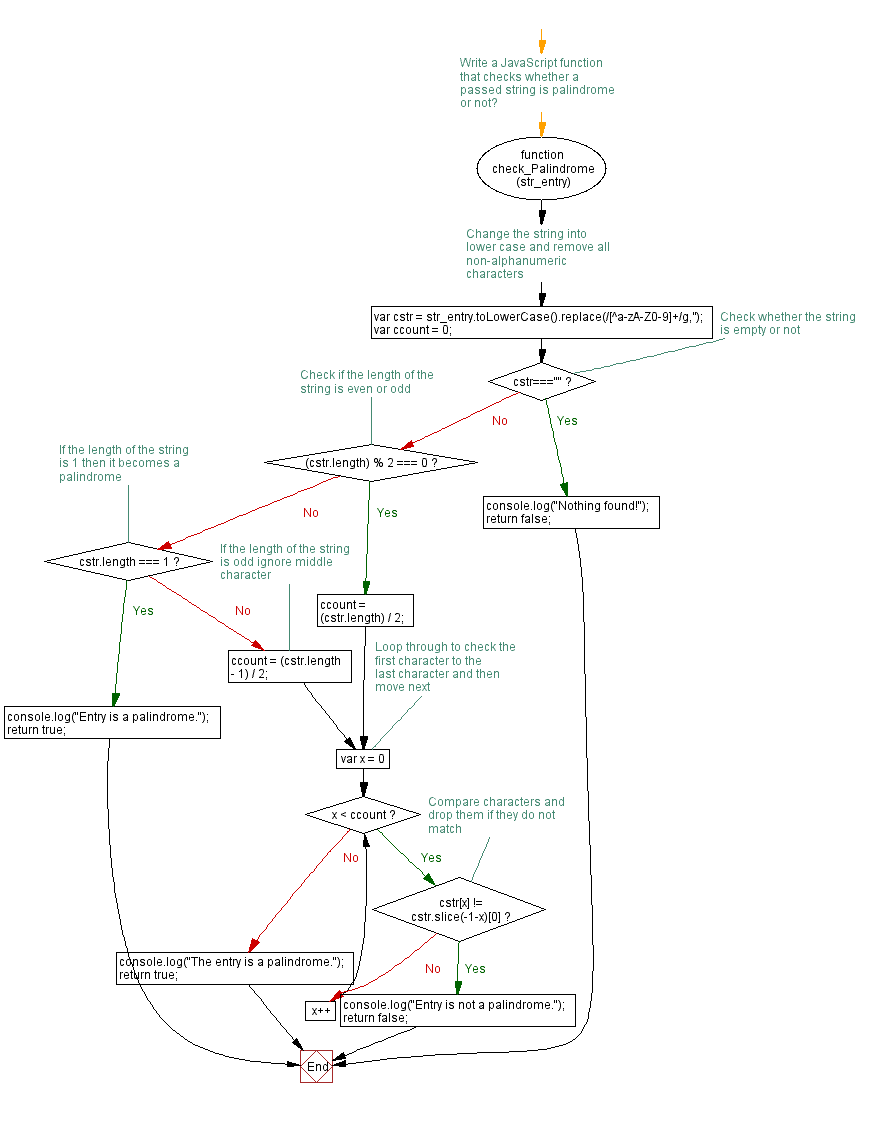
Live Demo:
See the Pen JavaScript -Check whether a passed string is palindrome or not-function-ex- 2 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Define a function named isPalindrome that takes a string parameter (str)
function isPalindrome(str) {
// Get the length of the string and store it in the constant variable len
const len = str.length;
// Iterate through the first half of the string
for (let i = 0; i < len / 2; i++) {
// Check if the character at position i is not equal to its corresponding character from the end
if (str[i] !== str[len - 1 - i]) {
// If not equal, return false as it is not a palindrome
return false;
}
}
// If the loop completes without returning false, the string is a palindrome, so return true
return true;
}
// Declare constant variables str1, str2, and str3 with different string values
const str1 = 'madam';
const str2 = 'nursesrun';
const str3 = 'fox';
// Call the isPalindrome function for each string and log the result to the console
console.log(isPalindrome(str1));
console.log(isPalindrome(str2));
console.log(isPalindrome(str3));
Output:
true true false
Explanation:
The above function compares the characters at the beginning and end of the string. If the characters do not match, the function returns false. If all the characters match, the function returns true
Flowchart:
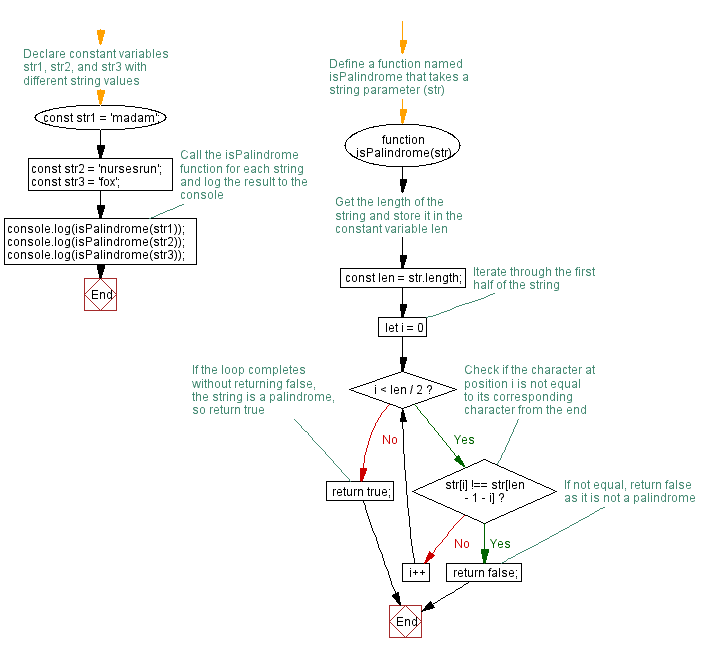
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that reverse a number.
Next: Write a JavaScript function that generates all combinations of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/javascript-function-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics