JavaScript: Generates all combinations of a string
JavaScript Function: Exercise-3 with Solution
String Combinations
Write a JavaScript function that generates all combinations of a string.
Example string: 'dog'
Expected Output: d,o,do,g,dg,og,dog
Visual Presentation:
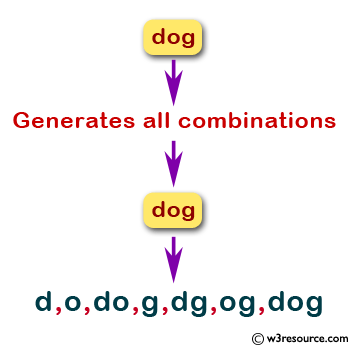
Sample Solution-1:
JavaScript Code:
// Write a JavaScript function that generates all combinations of a string.
function substrings(str1) {
// Initialize an empty array to store individual characters of the input string
var array1 = [];
// Loop through the characters of the input string and store each character in the array
for (var x = 0, y = 1; x < str1.length; x++, y++) {
array1[x] = str1.substring(x, y);
}
// Initialize an empty array to store all combinations
var combi = [];
var temp = "";
// Calculate the total number of combinations using the formula 2^n
var slent = Math.pow(2, array1.length);
// Generate all combinations using bitwise operations
for (var i = 0; i < slent; i++) {
temp = "";
// Iterate through each character in the array
for (var j = 0; j < array1.length; j++) {
// Check if the j-th bit of the binary representation of i is set
if (i & Math.pow(2, j)) {
// If set, append the corresponding character to the temporary string
temp += array1[j];
}
}
// If the temporary string is not empty, add it to the combinations array
if (temp !== "") {
combi.push(temp);
}
}
// Log the generated combinations, joined by newline, to the console
console.log(combi.join("\n"));
}
// Call the substrings function with the input string "dog"
substrings("dog");
Output:
d o do g dg og dog
Flowchart:
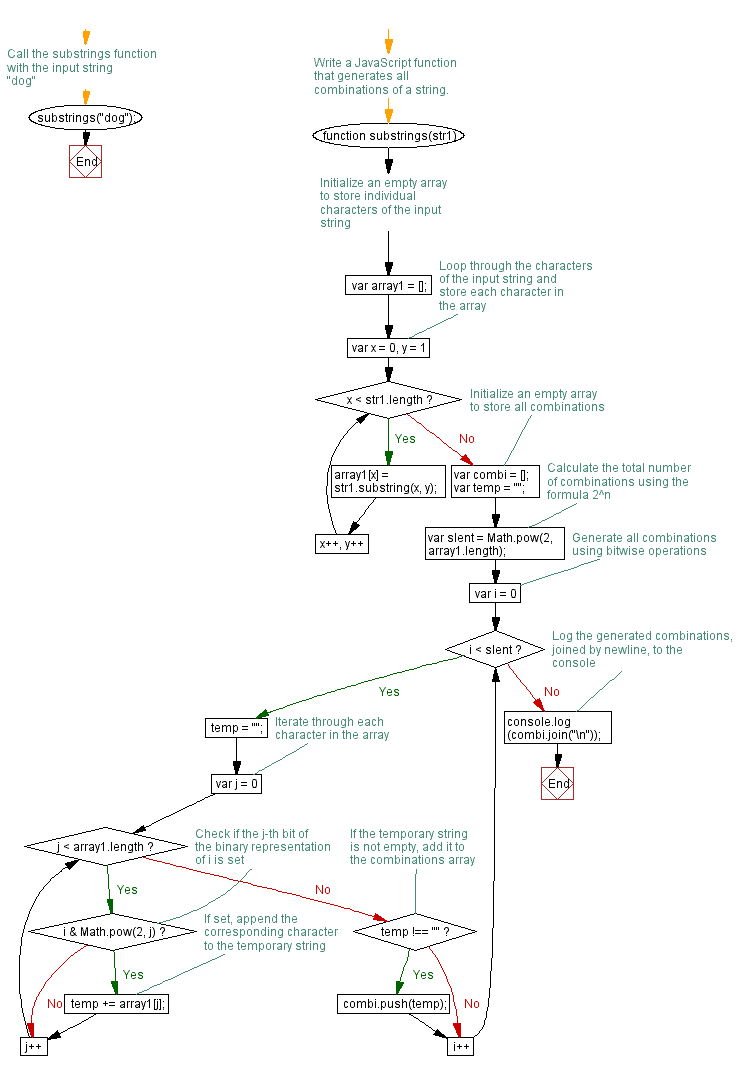
Live Demo:
See the Pen JavaScript -Check whether a passed string is palindrome or not-function-ex- 2 by w3resource (@w3resource) on CodePen.
Sample Solution-2:
JavaScript Code:
// Define a function named substrings that generates all combinations of a string
function substrings(str) {
// Initialize an array to store the result (combinations)
const result = [];
// Define a recursive helper function named search_combination
function search_combination(start, curr) {
// If the current combination is not empty, add it to the result array
if (curr.length > 0) {
result.push(curr);
}
// Iterate through the characters of the input string starting from the specified index
for (let i = start; i < str.length; i++) {
// Recursively call the search_combination function with updated parameters
search_combination(i + 1, curr + str[i]);
}
}
// Start the recursive search_combination function with initial parameters
search_combination(0, '');
// Return the array containing all combinations
return result;
}
// Declare a constant variable str with the value 'dog'
const str = 'dog';
// Call the substrings function with the input string 'dog' and store the result in the variable result
const result = substrings(str);
// Log the result (combinations) to the console
console.log(result);
Output:
["d","do","dog","dg","o","og","g"]
Explanation:
The above substrings() function takes a string as input and uses a function called search_combination () to recursively generate the combinations. The start parameter keeps track of the index in the input string where the function should start adding characters to the current combination, and the curr parameter stores the current combination being generated.
At each step, the function checks if the current combination is not empty and adds it to the result array if it is not. Then, the function loops over the remaining characters in the input string starting from the current index start, and recursively calls search_combination() function with the updated start and curr parameters. This generates all possible combinations of the characters in the string.
Flowchart:
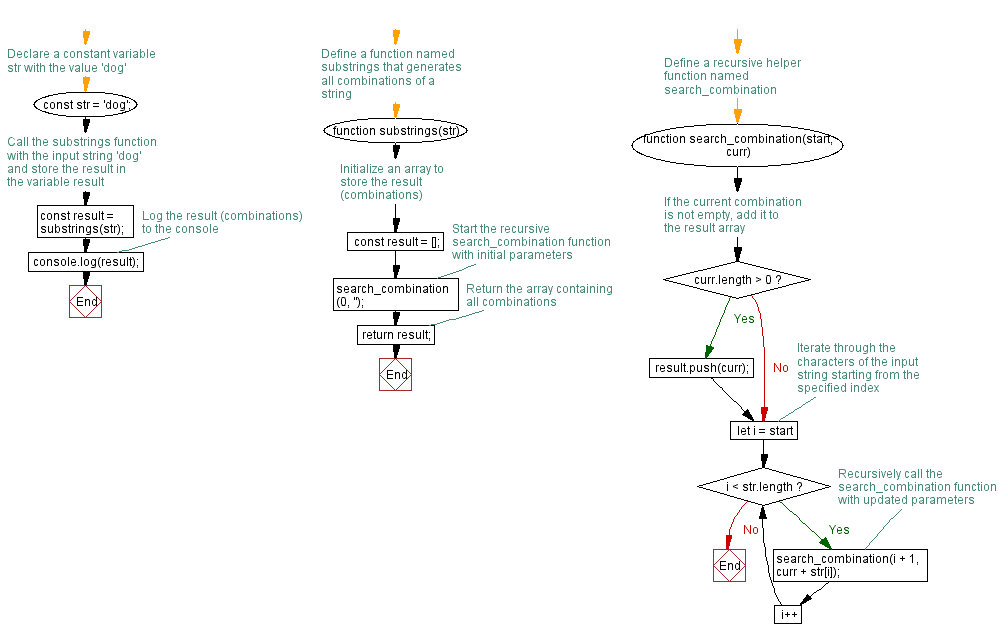
Live Demo:
See the Pen javascript-function-exercise-3-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript function that generates all permutations of a given string.
- Write a JavaScript function that returns all possible combinations of characters in a string, including the empty combination.
- Write a JavaScript function that produces all unique combinations from a string with duplicate characters.
- Write a JavaScript function that lists all substrings of a string and returns them in lexicographical order.
Improve this sample solution and post your code through Disqus.
Previous: Write a JavaScript function that checks whether a passed string is palindrome or not?
Next: Write a JavaScript function that returns a passed string with letters in alphabetical order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.