Python: Convert a string date to the timestamp
31. String Date to Timestamp
Write a Python program to convert a string date to a timestamp.
Sample Solution:
Python Code:
# Import the time module
import time
# Import the datetime module
import datetime
# Assign a date string to the variable 's'
s = "01/10/2016"
# Print an empty line
print()
# Convert the date string to a timestamp using datetime module and print it
print(time.mktime(datetime.datetime.strptime(s, "%d/%m/%Y").timetuple()))
# Print an empty line
print()
Output:
1494225605.0
Explanation:
In the exercise above,
- The code imports two modules: "time" and "datetime".
- It assigns a date string "01/10/2016" to the variable 's'. This string represents the date in the format of "day/month/year".
- Next, it converts the date string 's' into a timestamp using the "strptime()" method from the "datetime" module to parse the string into a datetime object, and then "mktime()" function from the "time" module to convert the datetime object into a Unix timestamp (the number of seconds since the Unix epoch - January 1, 1970).
- Finally, it prints the calculated timestamp.
Flowchart:
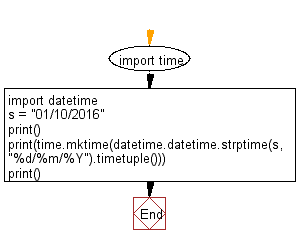
For more Practice: Solve these Related Problems:
- Write a Python program to parse a date string in "DD/MM/YYYY" format and then convert it to a Unix timestamp.
- Write a Python script that accepts a string date with time and returns its Unix timestamp after proper conversion.
- Write a Python function to convert a list of date strings into timestamps and then output the earliest and latest timestamps.
- Write a Python program to read a date string from input, convert it to a datetime object, and then print the corresponding timestamp.
Go to:
Previous: Write a Python program convert a date to timestamp.
Next: Write a Python program to calculate a number of days between two dates.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.