Python: Convert a date to timestamp
30. Date to Timestamp
Write a Python program to convert a date to a timestamp.
Sample Solution:
Python Code:
# Import the time module
import time
# Import the datetime module
import datetime
# Get the current date and time
now = datetime.datetime.now()
# Print the UNIX timestamp representing the current date and time
# The mktime function is used to convert the time tuple returned by now().timetuple() to a UNIX timestamp
print()
print(time.mktime(now.timetuple()))
print()
Output:
1494224813.0
Explanation:
In the exercise above,
- The code imports the "time" and "datetime" modules.
- Get the current date and time:
- It retrieves the current date and time using the "datetime.now()" method, which returns a 'datetime' object representing the current date and time.
- Converting to UNIX Timestamp:
- It converts the current date and time (now) to a UNIX timestamp using the "mktime()" function from the 'time' module.
- The "timetuple()" method of the 'datetime' object converts the 'datetime' object to a time tuple, which "mktime()" generates the UNIX timestamp.
- Finally it prints the UNIX timestamp representing the current date and time to the console.
Flowchart:
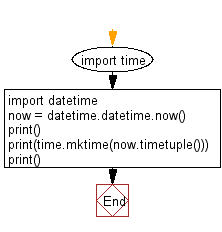
For more Practice: Solve these Related Problems:
- Write a Python program to convert a given date in "YYYY-MM-DD" format to a Unix timestamp and then print the result.
- Write a Python script that accepts a date from the user and returns its corresponding timestamp using the datetime module.
- Write a Python function to convert multiple dates to timestamps and then sort them based on the timestamp values.
- Write a Python program to take a date string, convert it to a datetime object, and then output the Unix timestamp.
Go to:
Previous: Write a Python program to get the GMT and local current time.
Next: Write a Python program convert a string date to the timestamp.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.