Python: Calculate number of days between two datetimes
33. Days Between DateTimes
Write a Python program to calculate the number of days between two date times.
Sample Solution:
Python Code:
# Import the datetime module
import datetime
# Import the datetime class from datetime module
from datetime import datetime
# Define a function called differ_days which calculates the difference in days between two datetime objects
def differ_days(date1, date2):
# Assign date1 to variable a
a = date1
# Assign date2 to variable b
b = date2
# Return the difference in days between the two datetime objects
return (a - b).days
# Print an empty line
print()
# Print the difference in days between October 12, 2016 (midnight) and December 10, 2015 (midnight)
print(differ_days((datetime(2016, 10, 12, 0, 0, 0)), datetime(2015, 12, 10, 0, 0, 0)))
# Print the difference in days between October 12, 2016 (midnight) and December 10, 2015 (11:59:59 PM)
print(differ_days((datetime(2016, 10, 12, 0, 0, 0)), datetime(2015, 12, 10, 23, 59, 59)))
# Print an empty line
print()
Output:
307 306
Explanation:
In the exercise above,
- It imports the "datetime" module.
- It defines a function named "differ_days()" that takes two datetime objects (date1 and date2) as input and returns the difference in days between them.
- Inside the function:
- It assigns the input datetime objects 'date1' and 'date2' to variables 'a' and 'b' respectively.
- It calculates the difference in days between the two datetime objects using the 'days' attribute of the "timedelta" object obtained by subtracting 'b' from 'a'.
- It returns the difference in days.
- Then, it calls the differ_days function twice:
- First, it calculates and prints the difference in days between October 12, 2016 (midnight) and December 10, 2015 (midnight).
- Second, it calculates and prints the difference in days between October 12, 2016 (midnight) and December 10, 2015 (11:59:59 PM).
Flowchart:
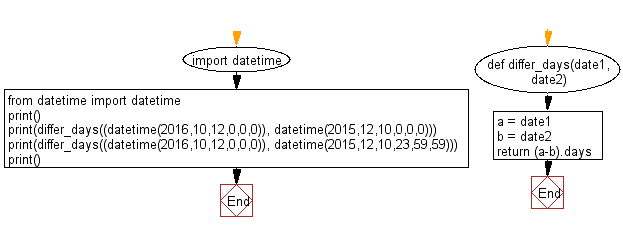
For more Practice: Solve these Related Problems:
- Write a Python program to compute the difference in days between two datetime values including hours, minutes, and seconds.
- Write a Python script to calculate the number of complete days between two datetime objects and then display the remainder hours.
- Write a Python function to determine the total number of days between two date-time strings and also return the difference in seconds.
- Write a Python program to find the gap between two datetime values and then output both the total days and the residual time in HH:MM:SS format.
Go to:
Previous: Write a Python program to calculate a number of days between two dates.
Next: Write a Python program to display the date and time in a human-friendly string.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.