Python PyQt keyboard event handling example
Python PyQt Event Handling: Exercise-3 with Solution
Write a Python program to build a PyQt application that responds to keyboard events such as key presses and releases.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QLabel Class: The QLabel widget provides a text or image display.
QWidget Class: The QWidget class is the base class of all user interface objects.
QKeyEvent Class: The QKeyEvent class describes a key event.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QWidget
from PyQt5.QtGui import QKeyEvent
class KeyboardEventApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Keyboard Event Example")
self.setGeometry(100, 100, 400, 200)
self.central_widget = QWidget(self)
self.setCentralWidget(self.central_widget)
self.key_label = QLabel("Last Key Pressed: None", self.central_widget)
self.key_label.setGeometry(10, 10, 200, 30)
def keyPressEvent(self, event):
if isinstance(event, QKeyEvent):
key_text = event.text()
self.key_label.setText(f"Last Key Pressed: {key_text}")
def keyReleaseEvent(self, event):
if isinstance(event, QKeyEvent):
key_text = event.text()
self.key_label.setText(f"Key Released: {key_text}")
def main():
app = QApplication(sys.argv)
window = KeyboardEventApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary PyQt5 modules.
- Create a custom class "KeyboardEventApp" that inherits from "QMainWindow".
- Inside the KeyboardEventApp class constructor (__init__), we set up the main window and a label to display keyboard events.
- Override the "keyPressEvent()" and "keyReleaseEvent()" methods to respond to key presses and releases, respectively. These methods are automatically called when keyboard events occur.
- Inside the "keyPressEvent()" and "keyReleaseEvent()" methods, we use event.text() to retrieve the text of the key that was pressed or released, and update the label with the corresponding information.
- Create an instance of the “KeyboardEventApp” class, show the main window, and start the PyQt5 application event loop.
Output:
Flowchart:
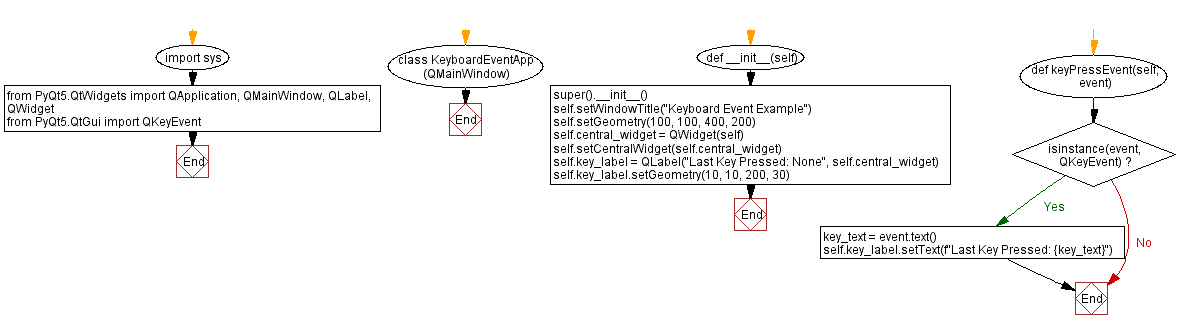
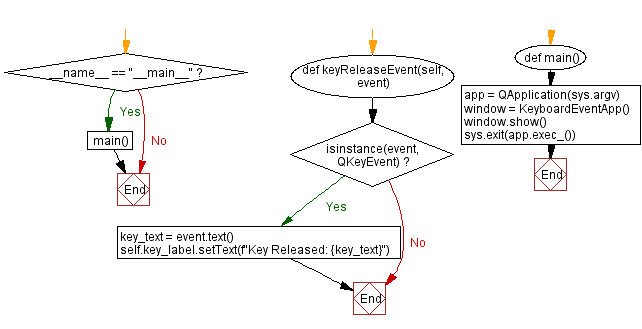
Python Code Editor:
Previous: Capture and display mouse events in PyQt5 with Python.
Next: Python PyQt custom button example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics