Swift Scripting: Downloading a schema
One of the convenience wrappers available to you in the target is ApolloSchemaDownloader. This allows you to use an ApolloSchemaOptions object to set up how you would like to download the schema.
1. Set up access to the endpoint you'll be downloading this from. This might be directly from your server, or from Apollo Graph Manager. For this example, let's download directly from the server:
let endpoint = URL(string: "http://localhost:8080/graphql")!
2. You will want to download your schema into the folder containing source files for your project:
Sample Project Structure
MyProject // SourceRoot
| MyProject.xcodeproj
| - MyProject // Contains app target source files
| schema.json
| - MyLibraryTarget // Contains lib target source files
| - MyProjectTests // Contains test files
| - Codegen // Contains Swift Scripting files
| Package.swift
| README.md
| - ApolloCLI // Contains downloaded typescript CLI
| - Sources
| - Codegen
| main.swift
To do that, set up the URL for the folder where you want to download the schema:
let output = sourceRootURL.apollo.childFolderURL(folderName:"MyProject")
Note that particularly if you're not just downloading the schema into your target's folder, you will want to make sure the folder exists before proceeding:
try FileManager
.default
.apollo.createFolderIfNeeded(at: output)
1. 1 Set up your ApolloSchemaOptions object. In this case, we'll use the default arguments for all the constructor parameters that take them, and only pass in the endpoint to download from and the folder to put the downloaded file into:
let schemaDownloadOptions = ApolloSchemaOptions(endpointURL: endpoint,
outputFolderURL: output)
With these defaults, this will download a JSON file called schema.json.
2. Add the code that will actually download the schema:
do {
try ApolloSchemaDownloader.run(with: cliFolderURL,
options: schemaDownloadOptions)
} catch {
exit(1)
}
Note that catching and manually calling exit with a non-zero code leaves you with a much more legible error message than simply letting the method throw.
- Build and run using the Xcode project. Note that if you're on Catalina you might get a warning asking if your executable can access files in a particular folder like this:
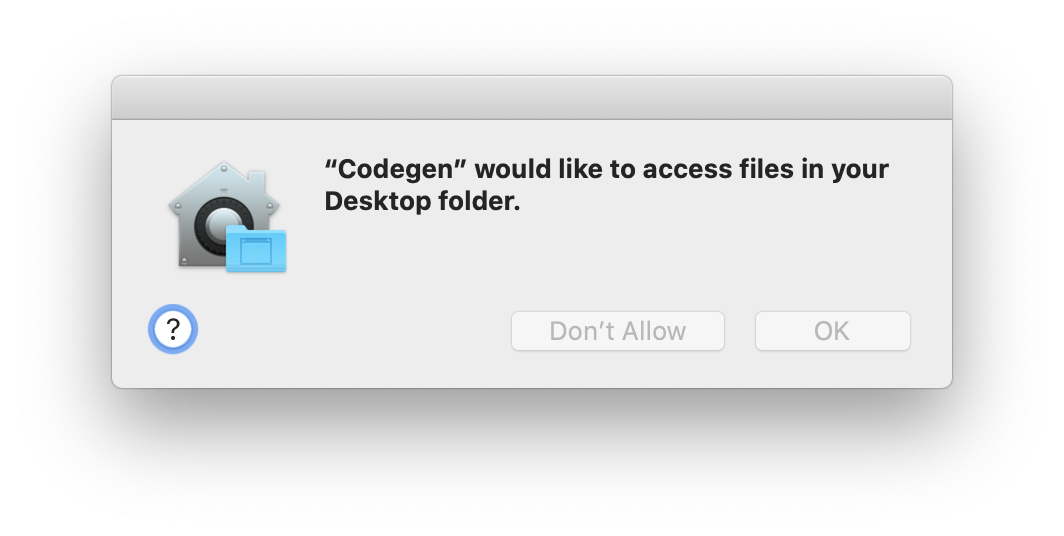
Click OK. Your CLI output will look something like this:
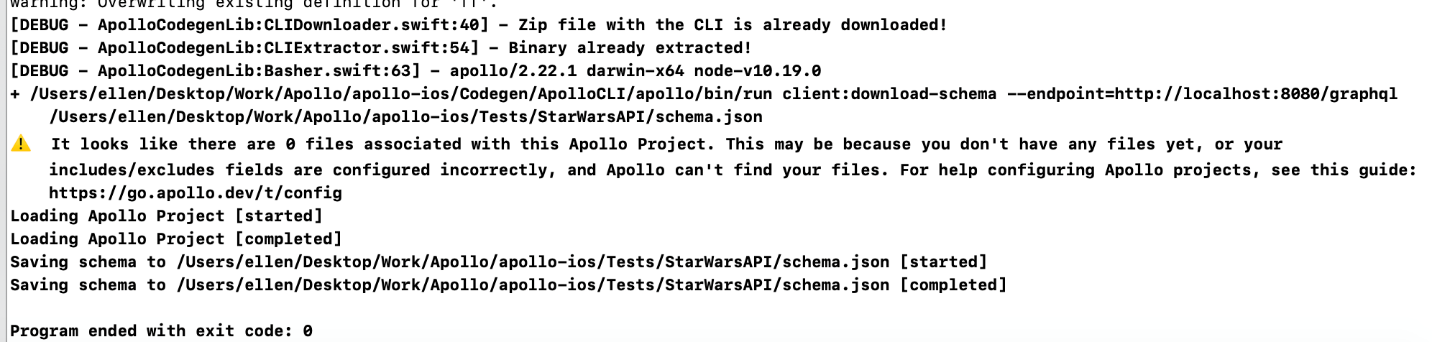
The last two lines (Saving schema started and Saving schema completed) indicate that the schema has successfully downloaded.
Note the warning: This isn't relevant for schema downloading, but it is relevant for generating code: In order to generate code, you need both the schema and some kind of operation.
Previous:
Composable networking for GraphQL
Next:
Concepts Overview
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics