C Exercises: Find the majority element of an array
33. Majority Element
Write a program in C to find the majority element of an array.
A majority element in an array A[] of size n is an element that appears more than n/2 times (and hence there is at most one such element).
The task requires writing a C program to identify the majority element in an array, which is defined as an element that appears more than half the time in the array. The program should count occurrences of each element and determine if any element meets this criterion, then output the result.
Visual Presentation:
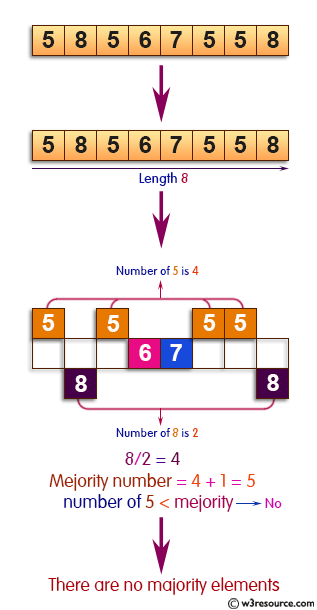
Sample Solution:
C Code:
#include <stdio.h>
// Function to find the majority element in an array
void findMajElem(int *arr1, int n) {
int i, IndexOfMajElem = 0, ctr = 1;
// Finding the candidate for the majority element
for (i = 1; i < n; i++) {
if (arr1[IndexOfMajElem] == arr1[i])
ctr++;
else
ctr--;
if (ctr == 0) {
IndexOfMajElem = i;
ctr = 1;
}
}
ctr = 0;
// Count occurrences of the candidate to confirm it as a majority element
for (i = 0; i < n; i++) {
if (arr1[i] == arr1[IndexOfMajElem])
ctr++;
}
// Checking if the candidate element occurs more than n/2 times
if (ctr > (n / 2))
printf("Majority Element : %d\n", arr1[IndexOfMajElem]);
else
printf("There are no Majority Elements in the given array.\n");
}
// Main function
int main() {
int i, ctr;
int arr1[] = { 4, 8, 4, 6, 7, 4, 4, 8 };
ctr = sizeof(arr1) / sizeof(arr1[0]);
// Display the given array
printf("The given array is : ");
for (i = 0; i < ctr; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
// Function call to find the majority element
findMajElem(arr1, ctr);
return 0;
}
Sample Output:
The given array is : 4 8 4 6 7 4 4 8 There are no Majority Elements in the given array.
Flowchart :
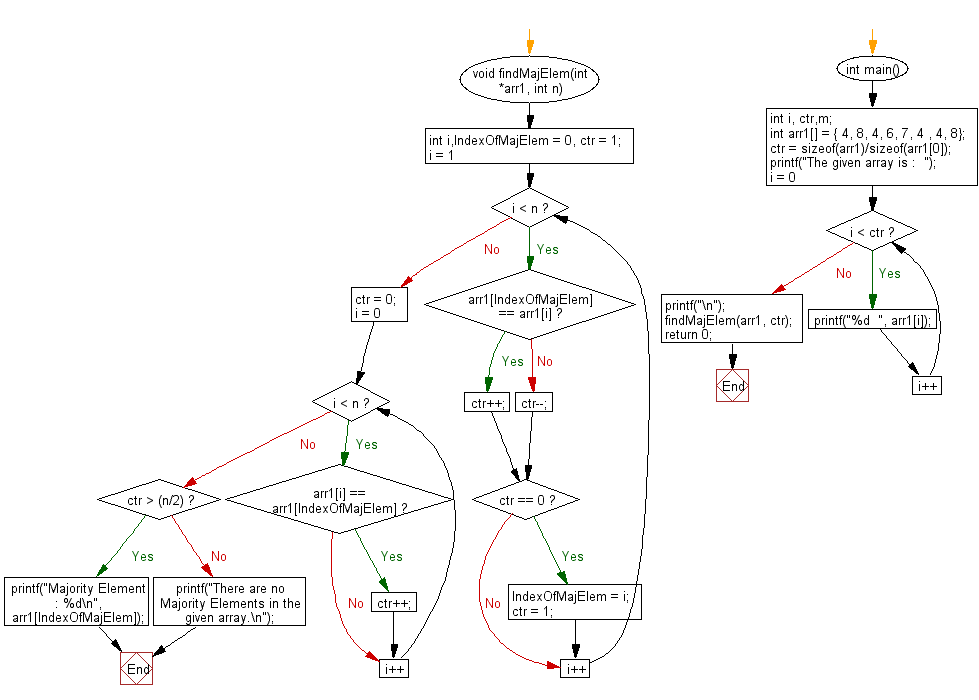
For more Practice: Solve these Related Problems:
- Write a C program to find the majority element using the Boyer-Moore voting algorithm.
- Write a C program to determine the majority element by first sorting the array and then scanning for duplicates.
- Write a C program to find the majority element recursively by dividing the array into halves.
- Write a C program to identify the majority element and then count its occurrences to validate the result.
C Programming Code Editor:
Previous: Write a program in C to find a pair with given sum in the array.
Next: Write a program in C to find the number occurring odd number of times in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.