C Exercises: Array fill, replace each subsequent position of the array by the double value of the previous
Replace first element of array and double subsequent values
Write a C program that reads an array of integers (length 7), and replaces the first element of the array by a given number and replaces each subsequent position of the array by the double value of the previous.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
int array_nums[7], i, x, k;
// Prompt user for the first element of the array
printf("Input the first element of the array:\n");
scanf("%d", &x);
// Loop to generate array elements by doubling 'x' in each iteration
for (k = 0, i = x; k < 7; i *= 2, k++) {
array_nums[k] = i; // Assign the calculated value to the array
}
// Print a message indicating the array elements will be displayed
printf("\nArray elements:\n");
// Loop to print each element of the array
for (i = 0; i < 7; i++) {
printf("array_nums[%d] = %d\n", i, array_nums[i]);
}
return 0; // End of program
}
Sample Output:
Input the first element of the array: 5 Array elements: array_nums[0] = 5 array_nums[1] = 10 array_nums[2] = 20 array_nums[3] = 40 array_nums[4] = 80 array_nums[5] = 160 array_nums[6] = 320
Flowchart:
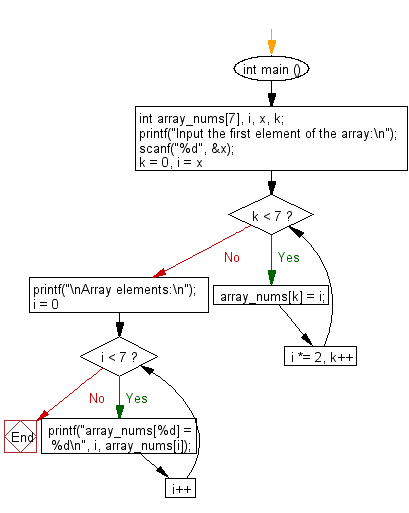
For more Practice: Solve these Related Problems:
- Write a C program to read an array and update it so that each element (except the first) becomes twice its previous element.
- Write a C program to perform in-place modification of an array where the first element is set and each following element is its double.
- Write a C program to use recursion to replace each element in an array with double the previous element starting from a given number.
- Write a C program to implement a function that updates an array such that every element after the first is the double of its predecessor.
C programming Code Editor:
Previous: Write a C program that reads an array of integers (length 7), replace every negative or null element by 1 and print the array elements.
Next: Write a C program that reads an array (length 7) and print all array positions that store a value less or equal to 0.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.