C Exercises: Select specific array values
Print positions of array elements
Write a C program that reads an array (length 7) and prints all array positions that store a value less than or equal to 0.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
float n, array_nums[7];
int i;
// Prompt user for input
printf("Input 7 array elements:\n");
// Loop to read 7 float values from the user and store them in the array
for (i = 0; i < 7; i++) {
scanf("%f", &n);
array_nums[i] = n;
}
// Print a message indicating the positions with values less than or equal to 0
printf("\nArray positions that store a value less or equal to 0:\n");
// Loop to check and print positions with values less than or equal to 0
for (i = 0; i < 7; i++) {
if (array_nums[i] <= 0) {
printf("array_nums[%d] = %.1f\n", i, array_nums[i]);
}
}
return 0; // End of program
}
Sample Output:
Input 7 array elements: 15 23 37 65 20 -7 65 Array positions that store a value less or equal to 0: array_nums[5] = -7.0
Flowchart:
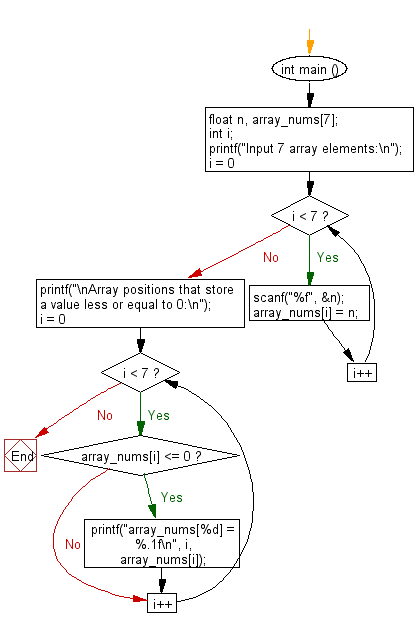
For more Practice: Solve these Related Problems:
- Write a C program to traverse an array and print indices and values of elements that are less than or equal to zero.
- Write a C program to iterate through an array and use conditional statements to display positions of non-positive elements.
- Write a C program to scan an array and output the index of each element meeting the condition (≤ 0) using pointer arithmetic.
- Write a C program to create an array, then filter and print positions where the stored value is zero or negative.
C programming Code Editor:
Previous: Write a C program that reads an array of integers (length 7), and replace the first element of the array by a give number and replace each subsequent position of the array by the double value of the previous.
Next: Write a C program that reads an array of integers (length 8), replace the 1st element by the 8th, 2nd by the 7th and so on. Print the final array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.