C Exercises: Change the array elements
Reverse the first and last elements of an array
Write a C program that reads an array of integers (length 8), replaces the 1st element with the 8th, the 2nd with the 7th and so on. Print the final array.
Sample Solution:
C Code:
#include <stdio.h>
int main () {
unsigned short i, j; // Declare variables for looping
short array_nums[8], n, temp1, temp2; // Declare array and temporary variables
// Prompt user for input
printf("Input 8 array elements:\n");
// Loop to read 8 short values from the user and store them in the array
for (i = 0; i < 8; i++) {
scanf("%hd", &n);
array_nums[i] = n;
}
// Loop to swap elements from the first half of the array with the second half
for (i = 0, j = 7; i <= 4 && j >= 4; i++, j--) {
temp1 = array_nums[i];
temp2 = array_nums[j];
array_nums[i] = temp2;
array_nums[j] = temp1;
}
// Print modified array
printf("\nModified array:\n");
for (i = 0; i < 8; i++)
printf("array_nums[%d] = %d\n", i, array_nums[i]);
return 0; // End of program
}
Sample Output:
Input 8 array elements: 25 35 17 -5 29 45 60 65 Modified array: array_nums[0] = 65 array_nums[1] = 60 array_nums[2] = 45 array_nums[3] = 29 array_nums[4] = -5 array_nums[5] = 17 array_nums[6] = 35 array_nums[7] = 25
Flowchart:
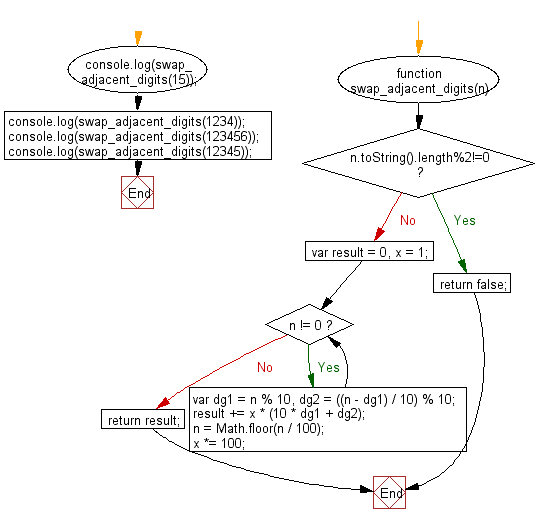
For more Practice: Solve these Related Problems:
- Write a C program to reverse an array in place by swapping elements from the ends towards the center.
- Write a C program to use a two-pointer technique to swap the first and last elements, second and second last, and so on.
- Write a C program to reverse the order of an array using recursion and print the modified array.
- Write a C program to create a function that reverses an array and demonstrate its use on an 8-element array.
C programming Code Editor:
Previous: Write a C program that reads an array (length 7) and print all array positions that store a value less or equal to 0.
Next: Write a C program that reads an array of integers (length 10), fill the array elements with number o to a (given number) n – 1 repeated times where 2 <= n <=10.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.