C Exercises: Print all prime factors of a given number
25. Prime Factorization Variants
Write a C program to print all prime factors of a given number.
Example:
Input: n = 75
Output: All prime factors of 75 are: 3 5 5
Visual Presentation:
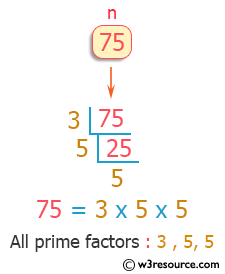
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Main function
int main(void) {
// Declare and initialize the variable 'n' to find its prime factors
int n = 75; // The number for which prime factors are to be found
printf("All prime factors of %d are: ", n); // Display the original number
// Check if the number 'n' is greater than 0 before finding its prime factors
if (n > 0) {
// Extract all the factors of 2
while (n % 2 == 0) {
printf("2 "); // Print '2' as a prime factor
n /= 2; // Divide 'n' by 2
}
// Check for odd factors starting from 3
for (int i = 3; i <= sqrt(n); i += 2) {
// Check if 'i' is a factor of 'n'
while (n % i == 0) {
printf("%d ", i); // Print 'i' as a prime factor
n /= i; // Divide 'n' by 'i'
}
}
// If 'n' is greater than 2 and has a prime factor > sqrt(n)
if (n > 2) {
printf("%d ", n); // Print 'n' as a prime factor
}
}
return 0; // End of the main function
}
Sample Output:
All prime factors of 75 are: 3 5 5
Flowchart:
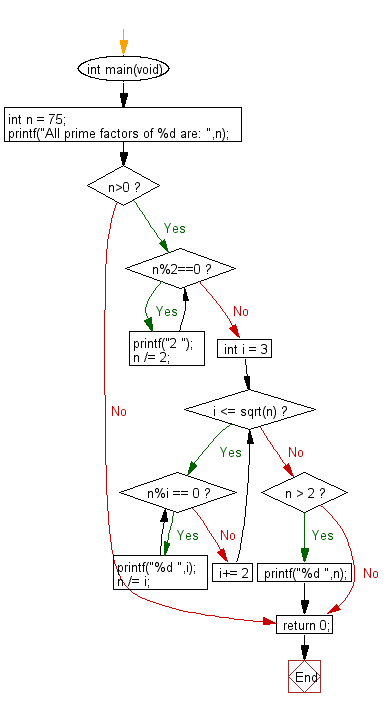
For more Practice: Solve these Related Problems:
- Write a C program to print all prime factors of a given number using trial division.
- Write a C program to factorize a number and display repeated prime factors with their counts.
- Write a C program to implement prime factorization using recursion and optimized trial division.
- Write a C program to compute the prime factors and then reconstruct the original number from those factors.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to calculate e raise to the power x using sum of first n terms of Taylor Series.
Next: Write a C program to check if a given number is Fibonacci number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.