C Exercises: Check if a given number is Fibonacci number or not
26. Fibonacci Number Check Variants
Write a C program to check if a given number is a Fibonacci number or not.
In mathematics, the Fibonacci numbers, commonly denoted Fn form a sequence, called the Fibonacci sequence, such that each number is the sum of the two preceding ones, starting from 0 and 1. That is, and for n > 1. By starting with 1 and 2, the first 10 terms will be: 1, 2, 3, 5, 8, 13, 21, 34, 55, 89.
Example:
Input: n = 8
Output: Is 8 a Fibonacci number? 1
Visual Presentation:
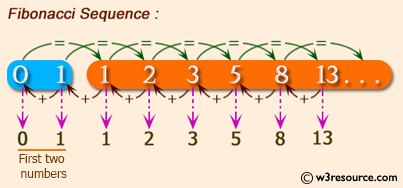
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
// Function to check if a number is a perfect square
int isPerfectSquare(int x) {
int s = (int)sqrt(x); // Calculate the square root of x and truncate to an integer
return (s * s == x); // Return true if x is a perfect square (if s*s is equal to x)
}
// Function to check if a number is a Fibonacci number
int isFibonacci(int x) {
// Check if the given number is a perfect square of (5 * x^2 + 4) or (5 * x^2 - 4)
return isPerfectSquare(5 * x * x + 4) || isPerfectSquare(5 * x * x - 4);
}
// Main function
int main(void) {
// Declare and initialize the number 'n' to check if it's a Fibonacci number
int n = 8; // The number to be checked
// Check if 'n' is greater than 0 before proceeding
if (n > 0) {
// Print whether 'n' is a Fibonacci number or not by calling the 'isFibonacci' function
printf("Is %d a Fibonacci number? %d", n, isFibonacci(n));
}
return 0; // End of the main function
}
Sample Output:
Is 8 a Fibonacci number? 1
Flowchart:
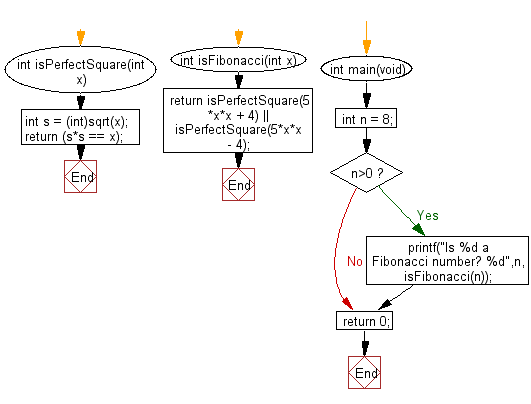
For more Practice: Solve these Related Problems:
- Write a C program to determine if a given number is a Fibonacci number using mathematical properties.
- Write a C program to check Fibonacci membership by generating Fibonacci numbers until the target is reached or exceeded.
- Write a C program to verify if a number is Fibonacci by testing if one of (5*n*n+4) or (5*n*n-4) is a perfect square.
- Write a C program to use recursion to generate Fibonacci numbers and then check if the given number is in the sequence.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to print all prime factors of a given number.
Next: Write a C program to multiply two numbers using bitwise operators.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.