C Exercises: Multiply two numbers using bitwise operators
27. Multiply Using Bitwise Operators Variants
Write a C program to multiply two numbers using bitwise operators.
Example:
Input: int x = 8
int y = 9
Output: Product of 8 and 9 using bitwise operators is: 72
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
// Function to perform multiplication using bitwise operators
int bitwise_multiply(int x, int y) {
// Adjust signs if both x and y are negative or if y is negative
if (x < 0 && y < 0) {
x = x * (-1);
y = y * (-1);
} else if (x > 0 && y < 0) {
x = x * (-1);
y = y * (-1);
}
int result = 0; // Initialize the result variable to store the product
// Multiply using bitwise operations
while (y > 0) {
if ((y & 1) != 0) // If the least significant bit of y is set
result = result + x; // Add x to the result
x = x << 1; // Left shift x to multiply it by 2
y = y >> 1; // Right shift y to divide it by 2
}
return result; // Return the final product
}
int main(void) {
int x = 8; // First number for multiplication
int y = 9; // Second number for multiplication
// Print the product of x and y calculated using bitwise operators
printf("Product of %d and %d using bitwise operators is: %d", x, y, bitwise_multiply(x, y));
return 0; // End of the main function
}
Sample Output:
Product of 8 and 9 using bitwise operators is: 72
Flowchart:
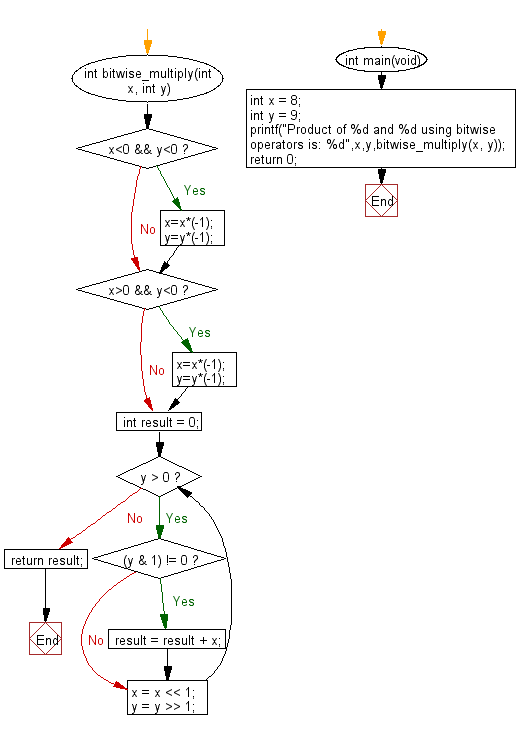
For more Practice: Solve these Related Problems:
- Write a C program to multiply two numbers using only bitwise operators and addition.
- Write a C program to simulate multiplication by shifting and adding, without using arithmetic operators.
- Write a C program to implement a bitwise multiplication algorithm and test it with both positive and negative numbers.
- Write a C program to multiply two integers using bitwise left shifts for doubling and recursive addition.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to check if a given number is Fibonacci number or not.
Next: Write a C program to find angle between given hour and minute hands.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.