C Exercises: Find angle between given hour and minute hands
C Programming Mathematics: Exercise-28 with Solution
Write a C program to find the angle between the hour and minute hands.
Example:
Input: int ha = 11
int ma = 30
Output: Angle between hour and minute hands 165
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
// Function to calculate the angle between the hour and minute hands of a clock
int calcAngle(int ha, int ma) {
// Adjusting hour and minute values to avoid errors for ha = 12 and ma = 60
if (ha == 12)
ha = 0;
if (ma == 60)
ma = 0;
// Calculate hour and minute angles based on clock readings
int hour_angle = (int)(0.5 * (ha * 60 + ma)); // Hour hand angle
int minute_angle = (int)(6 * ma); // Minute hand angle
// Calculate the absolute difference between hour and minute angles
int angle = abs(hour_angle - minute_angle);
// Calculate the smaller angle between the two possible angles
int complementary_angle = 360 - angle; // Calculate complementary angle
return (complementary_angle > angle) ? angle : complementary_angle;
}
int main(void) {
int ha = 11; // Hour hand position
printf("\nAngles move by hour hand: %d", ha);
int ma = 30; // Minute hand position
printf("\nAngles move by minute hand: %d", ma);
// Check for invalid inputs and display an error message if detected
if (ha < 0 || ma < 0 || ha > 12 || ma > 60) {
printf("\nWrong input..!");
} else {
// Calculate and display the angle between hour and minute hands
printf("\nAngle between hour and minute hands: %d", calcAngle(ha, ma));
}
return 0;
}
Sample Output:
Angles move by hour hand: 11 Angles move by minute hand: 30 Angle between hour and minute hands 165
Flowchart:
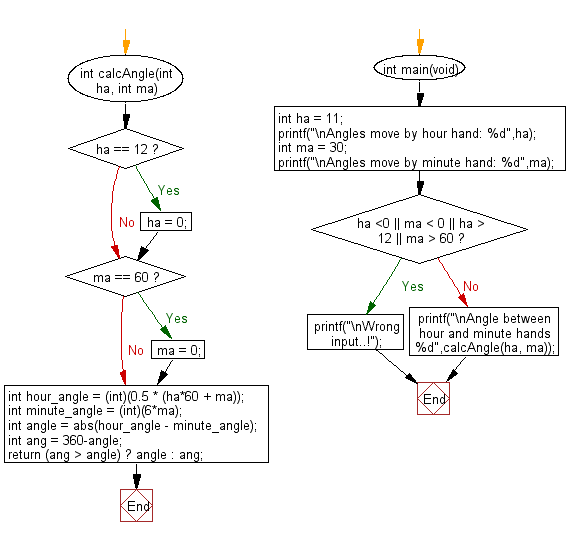
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Multiply two numbers using bitwise operators.
Next: Perfect square.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics