C Programming: Perfect square
29. Minimal Sum of Squares Variants
Write a C programming to get the smallest number of square numbers that add up to an integer n.
In mathematics, a perfect square is a number that can be expressed as either the product of an integer by itself or as the second exponent of an integer..
Sample Data:
14 = 32 + 22 + 12
Output – 3
15 = 32 + 22 + 12 + 12
Output - 4
16 = 42
Output – 1
17 = 42 + 12
Output – 2
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function to find the number of square numbers required to sum up to 'n'
int test(int n) {
// Allocate memory to store the minimum number of square numbers to sum up to 'n'
int *nums = (int *)malloc((n + 1) * sizeof(int));
// Initialize the allocated memory to a large value (here, 10000)
memset(nums, 10000, (n + 1) * sizeof(int));
// Base case: number of square numbers to sum to 0 is 0
nums[0] = 0;
// Dynamic programming approach to find the minimum number of square numbers needed
for (int i = 1; i <= n; i++) {
for (int j = 1; j * j <= i; j++) {
// Update nums[i] with the minimum of the current value and nums[i - j*j] + 1
nums[i] = nums[i] < nums[i - j * j] + 1 ? nums[i] : nums[i - j * j] + 1;
}
}
// Return the minimum number of square numbers required to sum up to 'n'
return nums[n];
}
int main(void) {
int n = 14;
printf("%d number of square numbers equal to %d", test(n), n);
n = 15;
printf("\n%d number of square numbers equal to %d", test(n), n);
n = 16;
printf("\n%d number of square numbers equal to %d", test(n), n);
n = 17;
printf("\n%d number of square numbers equal to %d", test(n), n);
return 0;
}
Sample Output:
3 number of square numbers equal to 14 4 number of square numbers equal to 15 1 number of square numbers equal to 16 2 number of square numbers equal to 17
Flowchart:
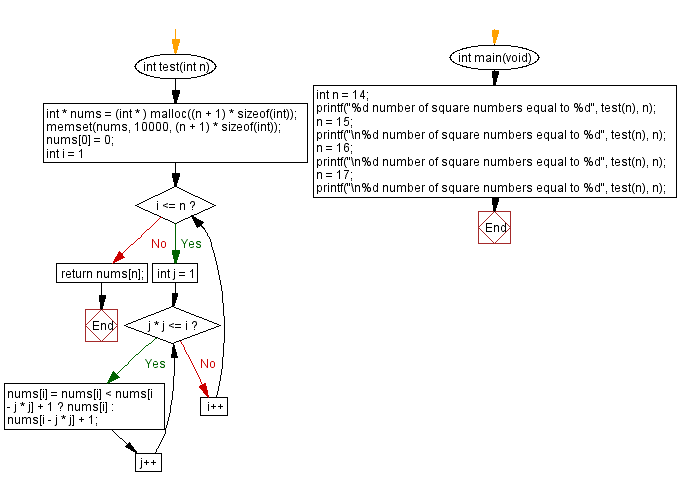
For more Practice: Solve these Related Problems:
- Write a C program to determine the minimum count of perfect squares that sum to a given integer using dynamic programming.
- Write a C program to compute the least number of square numbers needed to represent an integer using recursion with memoization.
- Write a C program to implement the optimal square-sum representation and output the squares used.
- Write a C program to find the minimum square sum decomposition by iterating over all square numbers less than n.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Find angle between given hour and minute hands.
Next: Count all the numbers with unique digits in a range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.