C Programming: Count all the numbers with unique digits in a range
30. Unique-Digit Numbers in Multiplication Table Variants
Write a C program that accepts a number (n) and counts all numbers with unique digits of length x within a specified range.
Range: 0 <= x < 10n
Example:
When n = 1, numbers with unique digits (10) between 0 and 9 are 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
When n = 2, numbers with unique digits (91) between 0 and 100 are 0,1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 12, 13, 14, 15 …..99 except 11, 22, 33, 44, 55, 66, 77, 88 and 99.
Test Data:
(1) -> 10
(2) -> 91
Sample Solution:
C Code:
#include <stdio.h> // Include standard input-output library
// Function to find the minimum of two numbers
int min(int a, int b){
return (a > b) ? b : a; // Return the smaller of the two numbers
}
// Function to calculate the number of unique digits in a range
int test(int n) {
if(n == 0)
return 1; // If n is 0, return 1 as there is only one unique digit (0)
n = min(10, n); // Limit n to be at most 10
if(n == 1)
return 10; // If n is 1, return 10 as there are 10 unique digits (0-9)
int flag = 9; // Initialize flag to 9
int result = 10; // Initialize result to 10
// Loop to calculate the number of unique digits in the range
for(int i = 2; i <= n; i++){
flag *= (9 - i + 2); // Update flag based on the number of remaining digits
result += flag; // Add the updated flag to the result
}
return result; // Return the total number of unique digits
}
int main(void) {
int n = 1; // Declare and initialize n to 1
printf("n = %d",n); // Print the value of n
printf("\nNumbers with unique digits in the range 0, 10: %d", test(n)); // Print the number of unique digits in the range 0 to 10
n = 2; // Update n to 2
printf("\n\nn = %d",n); // Print the value of n
printf("\nNumbers with unique digits in the range 0, 100: %d", test(n)); // Print the number of unique digits in the range 0 to 100
n = 3; // Update n to 3
printf("\n\nn = %d",n); // Print the value of n
printf("\nNumbers with unique digits in the range 0, 1000: %d", test(n)); // Print the number of unique digits in the range 0 to 1000
}
Sample Output:
n = 1 Numbers with unique digits in the range 0, 10: 10 n = 2 Numbers with unique digits in the range 0, 100: 91 n = 3 Numbers with unique digits in the range 0, 1000: 739
Flowchart:
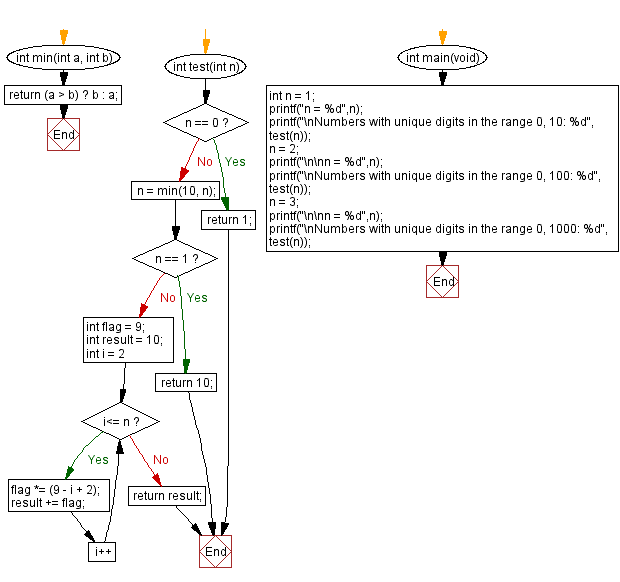
For more Practice: Solve these Related Problems:
- Write a C program to count numbers with all unique digits within a specified range using backtracking.
- Write a C program to generate numbers with unique digits of a given length and return their total count.
- Write a C program to verify uniqueness of digits for each number in a range without converting numbers to strings.
- Write a C program to list numbers with unique digits in a range and sort them in ascending order.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Perfect square.
Next: Largest number, swapping two digits in a number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.