C Programming: Largest number, swapping two digits in a number
31. Largest Number from One Swap Variants
Write a C programming to calculate the largest number that can be generated by swapping just two digits at most once
Test Data:
(89) -> 98 [Swapping 8 and 9]
(568) -> 865 [Swapping 8 and 5]
(4499) -> 9494 [Swapping 9 and 4]
(12345) -> 52341 [Swapping 1 and 5]
(7743) -> 52341 [No Swap]
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Function to swap two characters
void swap(char *x, char *y) {
char temp = *y; // Store the value of *y in temp
*y = *x; // Assign the value of *x to *y
*x = temp; // Assign the value of temp to *x
}
// Function to calculate the length of a string
int string_length(char *str)
{
int l = 0; // Declare and initialize variable l to 0
while(str[l] != '\0') // While loop to iterate through the string until the null terminator is encountered
{
l++; // Increment l
}
return l; // Return the length of the string
}
// Function to find the largest number by swapping two digits
int test(int num){
char str_num[1000]; // Declare an array to store the number as a string
char len; // Declare a variable to store the length of the string
int result; // Declare a variable to store the result
// Convert the integer to a string
sprintf(str_num, "%d", num);
// Get the length of the string
len = string_length(str_num);
// Initialize result to the input number
result = num;
// Nested loops to swap each pair of digits and find the maximum number
for (int i = 0; i < len; i++) {
for (int j = i+1; j < len; j++) {
swap(str_num+i, str_num+j); // Swap the digits at positions i and j in the string
if (atoi(str_num) > result) { // Convert the modified string to an integer and compare with the result
result = atoi(str_num); // Update the result if the modified number is greater
}
swap(str_num+i, str_num+j); // Restore the original order of digits
}
}
return result; // Return the largest number
}
int main(void) {
// Test cases
int n = 89;
printf("n = %d",n);
printf("\nLargest number from the said number swapping two digits = %d", test(n));
n = 568;
printf("\n\nn = %d",n);
printf("\nLargest number from the said number swapping two digits = %d", test(n));
n = 4499;
printf("\n\nn = %d",n);
printf("\nLargest number from the said number swapping two digits = %d", test(n));
n = 12345;
printf("\n\nn = %d",n);
printf("\nLargest number from the said number swapping two digits = %d", test(n));
n = 7743;
printf("\n\nn = %d",n);
printf("\nLargest number from the said number swapping two digits = %d", test(n));
}
Sample Output:
n = 89 Largest number from the said number swapping two digits = 98 n = 568 Largest number from the said number swapping two digits = 865 n = 4499 Largest number from the said number swapping two digits = 9494 n = 12345 Largest number from the said number swapping two digits = 52341 n = 7743 Largest number from the said number swapping two digits = 7743
Flowchart:
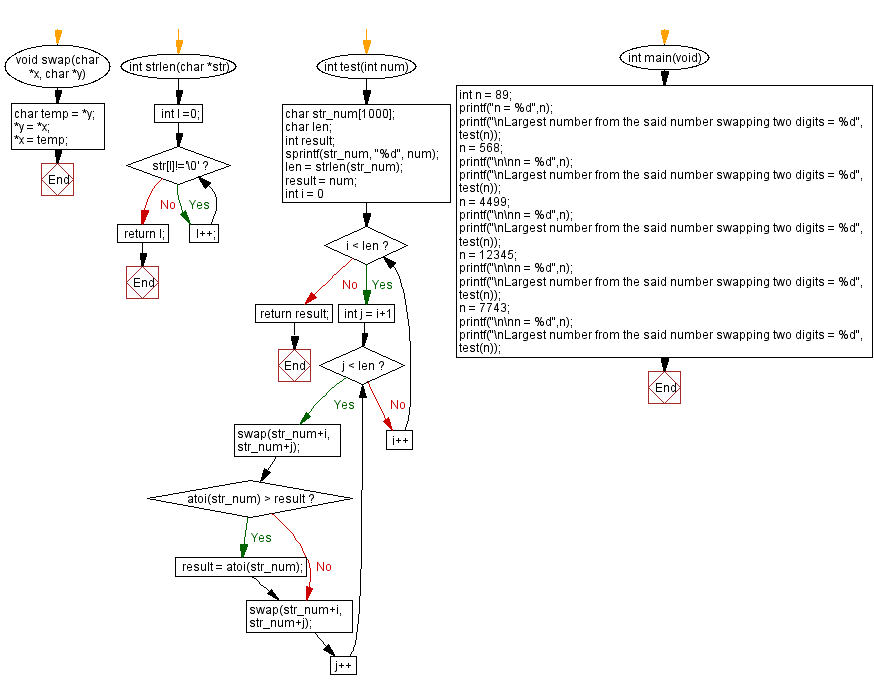
For more Practice: Solve these Related Problems:
- Write a C program to generate the largest number possible by swapping two digits at most once using brute-force.
- Write a C program to optimize the largest number generation by identifying the first pair that increases the number when swapped.
- Write a C program to determine the maximum number obtainable from one swap and validate with multiple test cases.
- Write a C program to simulate digit swapping and backtracking to ensure the optimal swap is performed.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Count all the numbers with unique digits in a range.
Next: Number sums and their reverses.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.