C Programming: Count unique digits of integers
37. Count Unique Digit Numbers Variants
Write a C program that counts the number of integers whose digits are unique from 1 and a given integer value.
Example:
Input: n = 30
From 1 to 30 all the integers have unique digits, except 11 and 22
Output: 28
Test Data:
(30) -> 28
(135) -> 110
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
// Function to count unique digits in an integer 'n'
int test(int n) {
// Calculate the length of the integer 'n'
int len = floor(log10(abs(n))) + 1;
int temp, i;
int count = 0;
int data[10] = { 0 }; // Array to store occurrences of digits (0-9)
// Extract digits from 'n' and count their occurrences
while (n > 0) {
temp = n % 10;
n = n / 10;
data[temp]++;
}
// Count unique digits (digits that occurred exactly once)
for (i = 0; i < 10; ++i) {
if (data[i] == 1)
count++;
}
// Check if the count of unique digits equals the length of 'n'
if (count == len)
return len; // Return the length if all digits are unique
else
return 0; // Return 0 if not all digits are unique
}
// Main function
int main(void) {
int n = 135, ctr = 0, result;
// Loop through integers from 1 to 'n' to count unique digits using the 'test' function
for (int i = 1; i <= n; i++) {
result = test(i);
if (result > 0) {
ctr++; // Increment counter if unique digits are found
result = 0;
}
}
// Display the count of integers with unique digits in the specified range
printf("Unique digits of integers from 1 and %d = %d", n, ctr);
}
Sample Output:
Unique digits of integers from 1 and 135 = 110
Flowchart:
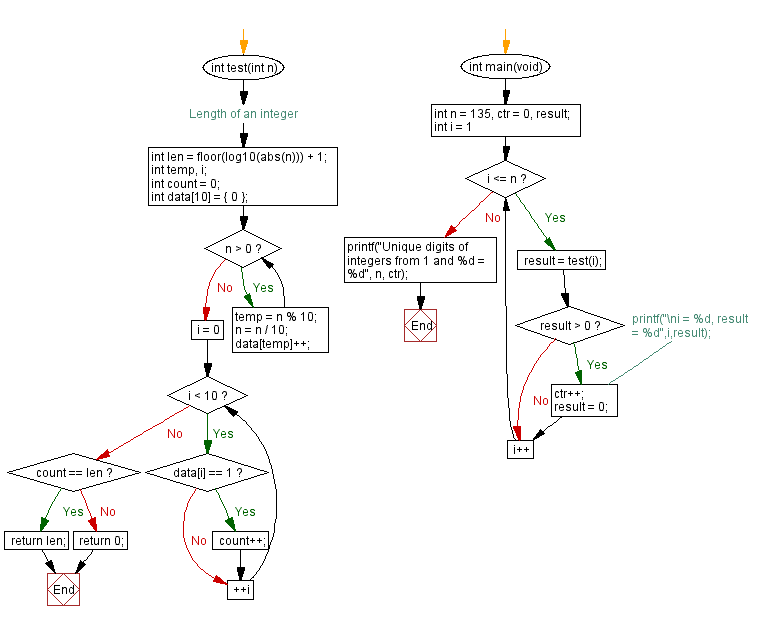
For more Practice: Solve these Related Problems:
- Write a C program to count numbers from 1 to n that have all unique digits using combinatorial methods.
- Write a C program to generate and count numbers with unique digits without converting them to strings.
- Write a C program to recursively determine if a number has unique digits and count how many do.
- Write a C program to list all numbers with unique digits in a given range and return the total count.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Count common factors of two integers.
Next: Count Integers with Odd digit sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.