C++ Exercises: Check a given array of integers and return true if every 5 that appears in the given array is next to another 5
Check if All '5's Are Next to Each Other
Write a C++ program to check a given array of integers and return true if every 5 that appears in the given array is next to another 5.
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Function to check if the number 5 is surrounded by another 5
static bool test(int numbers[], int arr_length)
{
bool flag = true; // Flag to indicate if a 5 is surrounded by another 5
// Loop through the array elements
for (int i = 0; i < arr_length; i++)
{
// Check for occurrences of 5
if (numbers[i] == 5)
{
// Condition to check if 5 is surrounded by another 5
if ((i > 0 && numbers[i - 1] == 5) || (i < arr_length - 1 && numbers[i + 1] == 5))
flag = true; // Set flag to true if 5 is surrounded by another 5
else if (i == arr_length - 1)
flag = false; // Set flag to false if the last element is 5 and it's not surrounded by another 5
else
return false; // Return false if 5 is not surrounded by another 5
}
}
return flag; // Return the flag indicating if 5 is surrounded by another 5 or not
}
// Main function
int main()
{
// Different test cases with arrays of integers
int nums1[] = {3, 5, 5, 3, 7}; // 5 is surrounded by another 5
int arr_length = sizeof(nums1) / sizeof(nums1[0]);
cout << test(nums1, arr_length) << endl;
int nums2[] = {3, 5, 5, 4, 1, 5, 7}; // 5 is not surrounded by another 5
arr_length = sizeof(nums2) / sizeof(nums2[0]);
cout << test(nums2, arr_length) << endl;
int nums3[] = {3, 5, 5, 5, 5, 5}; // 5 is surrounded by another 5
arr_length = sizeof(nums3) / sizeof(nums3[0]);
cout << test(nums3, arr_length) << endl;
int nums4[] = {2, 4, 5, 5, 6, 7, 5}; // 5 is not surrounded by another 5
arr_length = sizeof(nums4) / sizeof(nums4[0]);
cout << test(nums4, arr_length) << endl;
return 0; // Returning 0 to indicate successful completion of the program
}
Sample Output:
1 0 1 0
Visual Presentation:
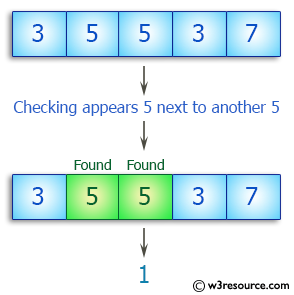
Flowchart:
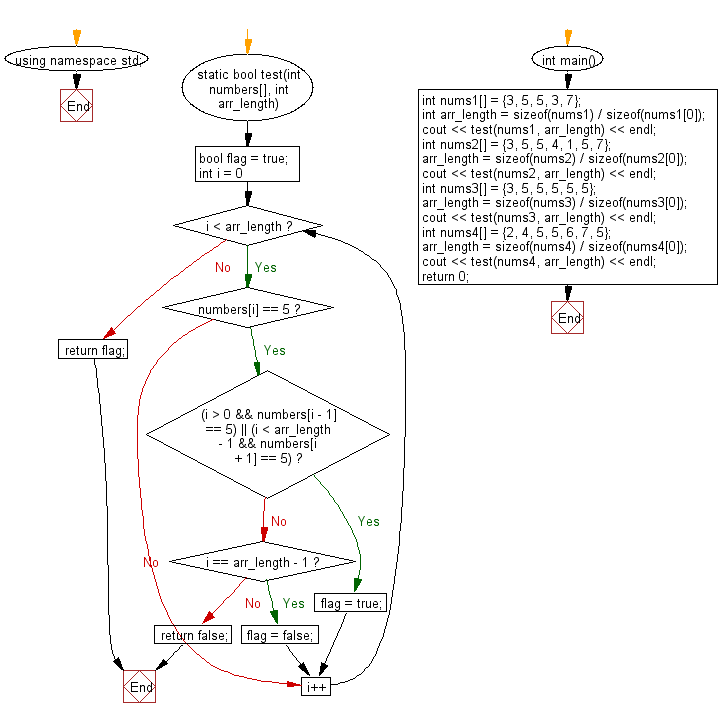
For more Practice: Solve these Related Problems:
- Write a C++ program to check if every occurrence of 5 in an array is adjacent to another 5, returning true if this condition holds.
- Write a C++ program that reads an integer array and outputs true if all 5's in the array appear in one contiguous block.
- Write a C++ program to determine if, in an array of integers, every 5 is immediately next to at least one other 5, then print the boolean result.
- Write a C++ program to scan an array and verify that 5's, if present, are grouped together, returning true if they are.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other.
Next: Write a C++ program to check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array.
What is the difficulty level of this exercise?