C++ String Exercises: Check if two characters present equally in a string
9. Check Equal Occurrence of Two Characters
Write a C++ program to check whether two characters appear equally in a given string.
Visual Presentation:
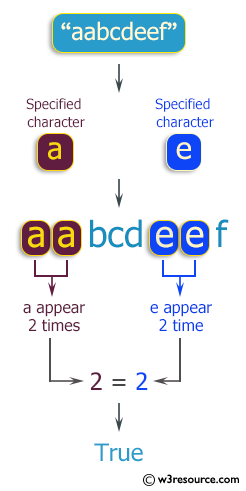
Sample Solution:
C++ Code :
#include <iostream> // Including input/output stream library
#include <string> // Including string handling library
using namespace std; // Using the standard namespace
// Function to count occurrences of two different characters in a string and check if their counts are equal
string count_chars(string str, string chr1, string chr2) {
int ctr1 = 0, ctr2 = 0; // Initializing counters for chr1 and chr2 occurrences
// Loop through the string to count occurrences of chr1 and chr2
for (int x = 0; x < str.length(); x++) {
if (str[x] == chr1[0]) // Checking if the current character matches chr1
ctr1++; // Increment counter for chr1 occurrences
if (str[x] == chr2[0]) // Checking if the current character matches chr2
ctr2++; // Increment counter for chr2 occurrences
}
// Check if the counts of chr1 and chr2 are equal and return True or False accordingly
if (ctr1 == ctr2) {
return "True"; // Return "True" if counts of chr1 and chr2 are equal
}
else {
return "False"; // Return "False" if counts of chr1 and chr2 are not equal
}
}
// Main function
int main() {
// Output the result of counting occurrences of characters in different strings
cout << count_chars("aabcdeef","a","e") << endl; // Output: True
cout << "\n" << count_chars("aabcdef","a","e") << endl; // Output: False
cout << "\n" << count_chars("aab11cde22f","1","2") << endl; // Output: True
return 0; // Return 0 to indicate successful completion
}
Sample Output:
True False True
Flowchart:
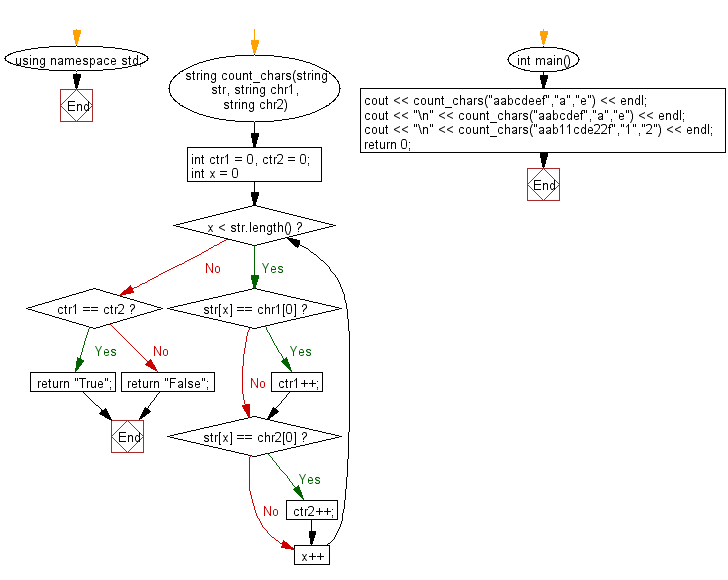
For more Practice: Solve these Related Problems:
- Write a C++ program to verify whether two specified characters occur equally often in a given string.
- Write a C++ program that reads a string and two characters, then compares their frequencies and outputs a boolean result.
- Write a C++ program to count the occurrences of two different letters in a string and check if their counts are equal.
- Write a C++ program to process an input string and return true if the frequency of one character matches that of another.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Count all the words in a given string.
Next C++ Exercise: Check if a given string is a Palindrome or not.
What is the difficulty level of this exercise?