Understanding the Array Data Structure: Characteristics & Operations
Array Data Structure:
In computer science, an array is a data structure consisting of a collection of elements (values or variables), of the same memory size, each identified by at least one array index or key. An array is stored such that the position of each element can be computed from its index tuple by a mathematical formula. The simplest type of data structure is a linear array, also called a one-dimensional array.
Here are some key characteristics and additional details:
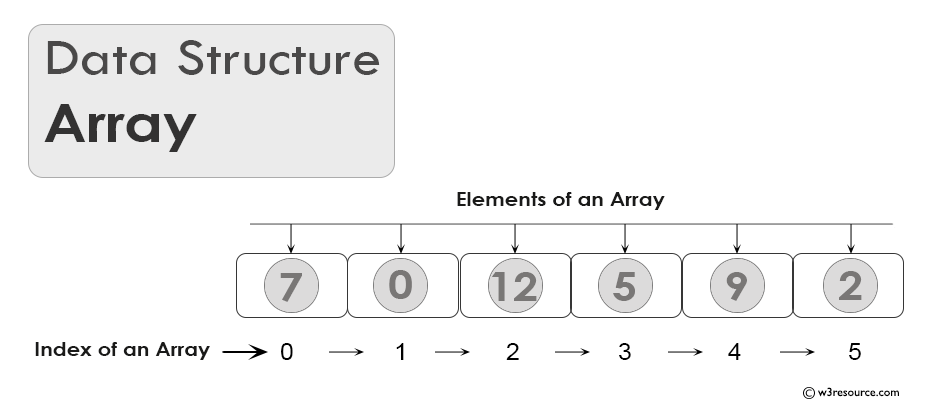
- Homogeneous elements:
- All elements in an array must be of the same data type. This ensures consistency and allows efficient memory allocation.
- Indexing:
- Elements in an array are accessed using their index, which is a numerical value representing their position in the array. Indexing typically starts at 0 in many programming languages, making the first element accessible with index 0, the second with index 1, and so on.
- Example (in Python):
# Create an integer array integer_array = [1, 2, 3, 4, 5]
- An array's size is usually fixed when declared. This fixed size is determined at the time of array creation and remains constant throughout its lifetime. Some programming languages may offer dynamic arrays or resizable arrays, allowing size adjustments during runtime.
- Arrays are memory-efficient as they store elements in contiguous memory locations. This contiguous storage enables quick and direct access to any element using its index. Memory efficiency is especially beneficial for large datasets.
- Arrays support various operations for efficient data manipulation, including:
- Insertion: Adding elements at a specific position or at the end of an array.
- Deletion: Removing elements from a specific position or based on their value.
- Update: Modifying the value of an existing element.
- Accessing Elements: Retrieving elements by their index.
- Traversal: Iterating through all array elements.
- Due to their contiguous memory allocation, arrays facilitate faster data retrieval compared to some other data structures. This is particularly advantageous when quick access to specific elements is essential.
- Some programming languages provide static arrays with a fixed size, while others offer dynamic arrays that can resize themselves during runtime to accommodate varying data requirements.
Limitations of arrays: Arrays have certain limitations that should be considered:
- Wasted Memory: Arrays may result in wasted memory if unused elements exist at the end. This occurs because arrays have a fixed size, and unused slots cannot be reclaimed for other purposes.
- Complexity of Insertion/Deletion: Inserting or deleting elements in the middle of an array can be complex. This is because shifting elements may be required to accommodate the new element, resulting in additional time and space complexity.
Real-world Example: Arrays find applications in various real-world scenarios
For instance, in a program tracking student grades, an array could be used to store the grades of each student. Each element of the array would represent the grade of a particular student, allowing easy access and manipulation of the data.
Similarly, in an e-commerce application, an array could store the prices of items. Each element of the array would represent the price of a specific item, enabling efficient management of product information.
Time Complexity: The time complexity of array operations varies:
- Random Access: Accessing elements in an array by index has a time complexity of O(1). This means that regardless of the size of the array, accessing any element takes constant time.
- Insertion/Deletion at Beginning/End: Inserting or deleting elements at the beginning or end of an array has a time complexity of O(1), amortized for dynamic arrays. This means that the time taken for these operations is constant on average, but occasional resizing may incur additional overhead.
- Insertion/Deletion in the Middle: Inserting or deleting elements in the middle of an array has a time complexity of O(n) in most cases. This is because shifting elements may be required to accommodate the new element, resulting in linear time complexity proportional to the size of the array.
- Dynamic Array: When we insert or delete elements at the beginning or end of dynamic arrays, it usually takes constant time (O(1)) on average. However, for static arrays, the time it takes might vary because they might need to copy all elements, which can be slower.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics