Learn Data Structures and Algorithms (DSA)
Here's a list of topics that beginners should know or learn before diving into Data Structures and Algorithms (DSA):
The Building Blocks of Technology: Understanding Algorithms
What are Data Structures and Algorithms (DSA)?
What is Data Structures?
From Wikipedia,
In computer science, a data structure is a data organization, management, and storage format that is usually chosen for efficient access to data. More precisely, a data structure is a collection of data values, the relationships among them, and the functions or operations that can be applied to the data, i.e., it is an algebraic structure about data.
Key characteristics:
- Organization: Data structures organize data to facilitate efficient access, modification, and storage.
- Operations: Each data structure supports specific operations, such as inserting, deleting, searching, and traversing elements.
- Memory Utilization: Data structures are designed to optimize memory usage, ensuring efficient storage and retrieval of information.
Types of Data Structures:
- Primitive Data Structures:
- Basic data types provided by programming languages (integers, floats, characters).
- Linear Data Structures:
- Elements are arranged sequentially, and each element has a unique predecessor and successor.
- Examples: Arrays, Linked Lists, Stacks, Queues.
- Non-Linear Data Structures:
- Elements are not arranged sequentially, and each element can have multiple predecessors and successors.
- Examples: Trees, Graphs.
- Homogeneous and Heterogeneous Data Structures:
- Homogeneous structures contain elements of the same data type.
- Heterogeneous structures can store elements of different data types.
Examples:
- Array:
- Linear data structures store elements of the same data type in contiguous memory locations.
- Linked List:
- Linear data structure where elements are stored in nodes, and each node points to the next one.
- Tree:
- Non-linear hierarchical data structure with a root node and branches of nodes, ending in leaves.
- Graph:
- Non-linear data structure consisting of nodes connected by edges, representing relationships between entities.
Note: Choice of data structure depends on the specific problem you're trying to solve.
Homogeneous Data Structures:
In homogeneous data structures, all elements are of the same data type. This uniformity simplifies data handling and allows consistent operations on the elements.
- Arrays:
- Elements are of the same data type (e.g., integers, floats).
- Example (in Python):
integer_array = [1, 2, 3, 4, 5]
- Elements are of the same data type.
- Example (in Python):
integer_list = [1, 2, 3, 4, 5]
- One-dimensional array-like structures with homogeneous elements.
- Example (in C++):
std::vector<int> integer_vector = {1, 2, 3, 4, 5};
Heterogeneous Data Structures:
Heterogeneous data structures have different data types. This flexibility allows for the storage of diverse information but may require additional handling for operations.
struct Person { char name[50]; int age; float height; };
- Structs (in some programming languages):
- Collection of different data types under a single structure.
- Example (in C):
struct Person { char name[50]; int age; float height; };
- Ordered collection of elements of different data types.
- Example (in Python):
person_info = ('John', 25, 1.75)
- Associative arrays with key-value pairs, where values can be of different data types.
- Example (in Python):
person_dict = {'name': 'John', 'age': 25, 'height': 1.75}
- Similar to Structs, allowing the grouping of different data types.
- Example (in Pascal):
type PersonRecord = record Name: String; Age: Integer; Height: Real; end;
The above examples illustrate the distinction between homogeneous and heterogeneous data structures based on the uniformity or diversity of data types within the structure. The choice between them depends on the specific requirements and characteristics of the data being handled.
The Building Blocks of Technology: Understanding Algorithms
In mathematics and computer science, an algorithm is a finite sequence of rigorous instructions, typically used to solve a class of specific problems or to perform a computation. Algorithms are used as specifications for performing calculations and data processing. More advanced algorithms can use conditionals to divert the code execution through various routes (referred to as automated decision-making) and deduce valid inferences (referred to as automated reasoning), achieving automation eventually.
Key characteristics:
- Preciseness: Algorithms are expressed in a clear and unambiguous manner, providing a precise sequence of steps to accomplish a task.
- Finiteness: Algorithms must terminate after a finite number of steps. They cannot go on indefinitely.
- Input: An algorithm receives input data, processes it through a sequence of well-defined steps, and produces output.
- Effectiveness: Algorithms are designed to be effective, meaning they should solve the problem for any valid input within a reasonable amount of time.
- Comprehensiveness: Algorithms are often designed with efficiency in mind (i.e., using minimal resources to achieve the desired outcome).
Components of an algorithm:
- Input: The data or values that the algorithm processes.
- Output: The result produced after the algorithm executes.
- Operations: Well-defined steps or actions that transform the input into the desired output.
- Control Structures: Structures such as loops and conditionals that determine execution flow.
Examples:
Non-technical example of an algorithm: In everyday life an algorithm for following a recipe involves steps like gathering ingredients, mixing them, and baking for a specific time.
Technical Example:
- Sorting Algorithm (e.g., QuickSort):
- Input: An unsorted list of elements.
- Output: Sorted list of elements.
- Operations: Comparison and swapping of elements based on a pivot value.
- Search algorithm (e.g., Binary Search):
- Input: Sorted list of elements, target element to find.
- Output: Index of the target element or indication of its absence.
- Operations: Repeatedly dividing the search interval in half based on comparisons.
- Graph Traversal (e.g., Depth-First Search):
- Input: Graph, starting vertex.
- Output: Traversal order of vertices.
- Operations: Visit and mark vertices in a systematic manner.
What are Data Structures and Algorithms (DSA)?
Data Structures and Algorithms (DSA) are foundational concepts in computer science that involve the study of organizing, storing, and manipulating data efficiently, as well as the design and analysis of algorithms to solve computational problems. DSA plays a crucial role in software development, enabling efficient and scalable solutions for a wide range of problems.
Key components:
- Data structures:
- Definition: Specialized formats for organizing and storing data to facilitate efficient operations.
- Purpose: Enable the effective management and retrieval of data by defining relationships between elements.
- Examples:
- Arrays: Efficient for storing a fixed-size collection of similar data types. They are particularly useful when you need random access to elements based on an index.
- Linked Lists: Linked lists are suited for situations where you need dynamic memory allocation, such as when the size of the data structure is unknown or frequently changing. They're efficient for insertion and deletion operations, but random access by index is slower than arrays.
- Trees: Trees are suited for hierarchical data storage, such as organizing hierarchical data like file systems or representing relationships like in family trees. They're also useful for data where parent-child relationships exist.
- Graphs: Graphs are suited for modeling relationships between pairs of objects. Useful for a wide range of applications, such as social networks, transportation networks, and computer networks.
- Hash Tables: A hash table, also known as a hash map or a hash set, is a data structure that implements an associative array, also called a dictionary, which is an abstract data type that maps keys to values. A hash table uses a hash function to compute an index, also called a hash code, into an array of buckets or slots, from which the desired value can be found
- Algorithms:
- Definition: Step-by-step procedures or sets of rules for solving a specific computational problem.
- Purpose: Provide systematic and efficient problem-solving approaches by defining a sequence of operations.
- Examples: Sorting Algorithms (e.g., QuickSort), Searching Algorithms (e.g., Binary Search), Graph Algorithms (e.g., Depth-First Search).
Relationship between Data Structures and Algorithms (DSA):
- Data structures provide a way to organize and store data.
- Algorithms define methods to manipulate and process data efficiently.
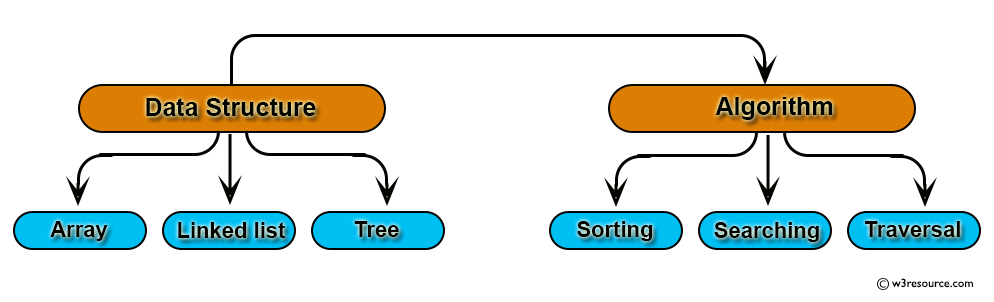
Importance of Data Structures and Algorithms (DSA):
- DSA helps create efficient algorithms, optimize resource usage, and improve program performance.
- Scalability: Well-designed data structures and algorithms enable software to handle larger datasets and scale to meet increasing demands.
- Problem-Solving: DSA provides a systematic approach to solving computational problems, fostering logical and structured thinking.
- Foundation for Programming: Understanding DSA is fundamental for designing and implementing software solutions in programming.
- Interviews and Assessments: DSA knowledge is often tested in technical interviews and coding assessments for job positions in the software industry.
- Optimized Software Development: DSA plays a critical role in creating correct software that is efficient and scalable.
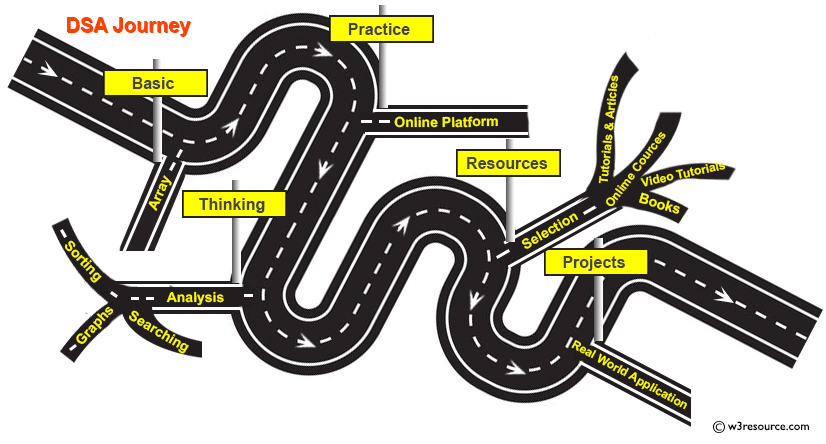
Here are some tips for learning DSA:
- Sequential Learning: Begin with the basics, such as arrays and basic algorithms, before moving on to more complex structures like trees and advanced algorithms.
- Practice: Gain proficiency through hands-on coding exercises, solving problems on online platforms, and participating in coding challenges.
- Algorithmic Thinking: Develop a mindset for algorithmic problem-solving, including breaking down problems, designing efficient algorithms, and analyzing their complexity.
- Resource Utilization: Learn to select appropriate data structures and algorithms based on problem requirements, emphasizing memory and time efficiency.
- Application in Projects: Apply DSA concepts to real-world projects to reinforce learning and gain practical experience.