Java: Display Pascal's triangle
Java Conditional Statement: Exercise-22 with Solution
Write a Java program to display Pascal's triangle.
Construction of Pascal's Triangle:
As shown in Pascal's triangle, each element is equal to the sum of the two numbers immediately above it.
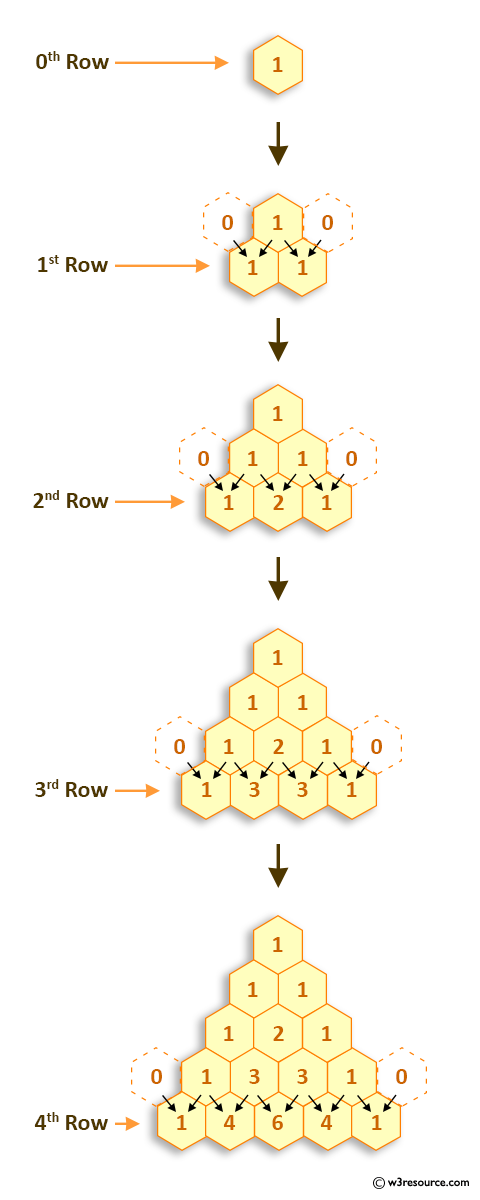
Test Data
Input number of rows: 5
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise22 {
public static void main(String[] args)
{
int no_row,c=1,blk,i,j;
System.out.print("Input number of rows: ");
Scanner in = new Scanner(System.in);
no_row = in.nextInt();
for(i=0;i<no_row;i++)
{
for(blk=1;blk<=no_row-i;blk++)
System.out.print(" ");
for(j=0;j<=i;j++)
{
if (j==0||i==0)
c=1;
else
c=c*(i-j+1)/j;
System.out.print(" "+c);
}
System.out.print("\n");
}
}
}
Sample Output:
Input number of rows: 5 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
Flowchart:
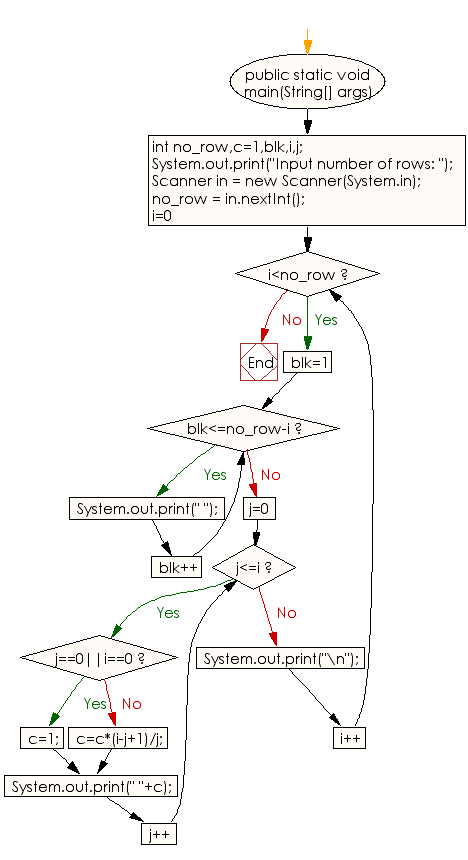
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in Java to display the pattern like a diamond.
Next: Write a java program to generate a following *'s triangle.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/conditional-statement/java-conditional-statement-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics