JavaFX Password match checker
JavaFx User Interface Components: Exercise-6 with Solution
Write a JavaFX program with two password fields. When the user enters a password in both fields and presses a button, check if the passwords match and display a message accordingly.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Password Match Checker");
// Create labels for password fields.
Label passwordLabel1 = new Label("Enter Password:");
Label passwordLabel2 = new Label("Confirm Password:");
// Create password input fields.
PasswordField passwordField1 = new PasswordField();
PasswordField passwordField2 = new PasswordField();
// Create a label to display the result.
Label resultLabel = new Label();
// Create a "Check Password" button.
Button checkButton = new Button("Check Password");
// Set an action for the button to check if passwords match.
checkButton.setOnAction(event -> {
String password1 = passwordField1.getText();
String password2 = passwordField2.getText();
if (password1.equals(password2)) {
resultLabel.setText("Passwords match!");
} else {
resultLabel.setText("Passwords do not match. Please check.");
}
});
// Create a layout (VBox) to arrange the elements.
VBox root = new VBox(10);
root.getChildren().addAll(passwordLabel1, passwordField1, passwordLabel2, passwordField2, checkButton, resultLabel);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.setTitle("Password Match Checker App");
// Show the window.
primaryStage.show();
}
}
In the above JavaFX exercise, there are two password fields, a "Check Password" button, and a label to display the result. When the user enters a password in both fields and clicks the button, it checks if the passwords match. It displays either "Passwords match!" or "Passwords do not match. Please check." The elements are organized using a 'VBox'.
Sample Output:
Flowchart:
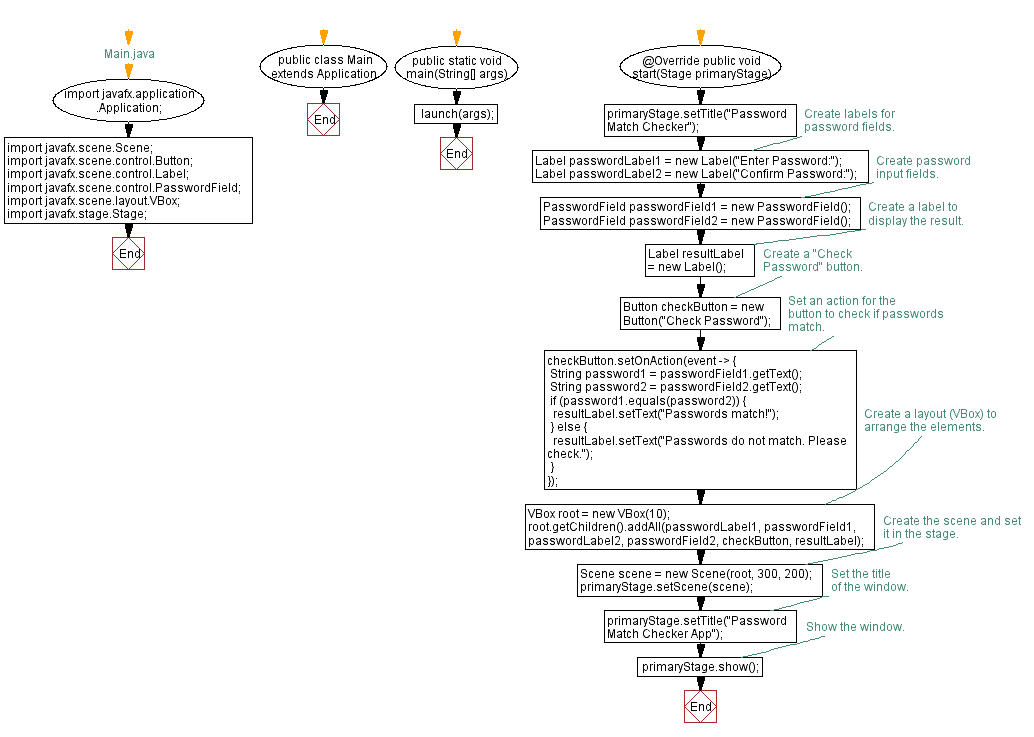
Java Code Editor:
Previous: JavaFX Login form application.
Next: JavaFX Color choice application.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-exercises/javafx/javafx-user-interface-components-exercise-6.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics