Java String: join() Method
join() Method
Contents:
public static String join(CharSequence delimiter, CharSequence... elements)
The join() method Returns a new String composed of copies of the CharSequence elements joined together with a copy of the specified delimiter.
Note that if an element is null, then "null" is added.
Java Platform: Java SE 8
Syntax:
join(CharSequence delimiter, CharSequence... elements)
Parameter:
Name | Description | Type |
---|---|---|
delimiter | the delimiter that separates each element | String |
elements | the elements to join together. | String |
Return Value: a new String that is composed of the elements separated by the delimiter
Return Value Type: String
Pictorial presentation of Java String join() Method
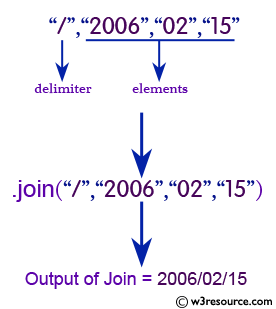
Example: Java String join(CharSequence delimiter, CharSequence... elements) Method
The following example shows the usage of java String() method.
import java.lang.*;
public class StringExample {
public static void main(String[] args) {
// join(CharSequence delimiter, CharSequence... elements)
String joined = String.join("-", "2006", "02", "15" );
System.out.println();
// prints length of string
System.out.println("Output of Join = " +joined);
System.out.println();
}
}
Output:
Output of Join = 2006-02-15
public static String join(CharSequence delimiter, Iterable<? extends CharSequence> elements)
Returns a new String composed of copies of the CharSequence elements joined together with a copy of the specified delimiter.
Note that if an individual element is null, then "null" is added.
Java Platform: Java SE 8
Syntax:
join(CharSequence delimiter, Iterable<? extends CharSequence> elements)
Parameter:
Name | Description | Type |
---|---|---|
delimiter | a sequence of characters that is used to separate each of the elements in the resulting String | String |
elements | an Iterable that will have its elements joined together. | String |
Return Value: a new String that is composed of the elements argument.
Return Value Type: String
Throws: NullPointerException - If delimiter or elements are null.
Example: Java String join(CharSequence delimiter, Iterable<? extends CharSequence> elements) Method
The following example shows the usage of java String() method.
import java.util.ArrayList;
import java.util.List;
public class ListJoin {
public static void main(String[] args) {
// join(CharSequence delimiter, Iterable<? extends CharSequence> elements)
List<String> strList = new ArrayList<String>();
strList.add("w3r");
strList.add("Join");
strList.add("Example");
// joining with comma as delimiter
String finalStr = String.join(";", strList);
System.out.println();
System.out.println("str - " + finalStr);
System.out.println();
}
}
Output:
str - w3r;Join;Example
Java Code Editor :
Previous:isEmpty Method
Next:lastIndexOf Method
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/java-tutorial/string/string_join.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics