Java String: startsWith() Method
public boolean startsWith(String prefix, int toffset)
The startsWith() method tests if the substring of this string beginning at the specified index starts with the specified prefix.
Java Platform: Java SE 8
Syntax:
startsWith(String prefix, int toffset)
Parameters:
Name | Description | Type |
---|---|---|
prefix | the prefix. | String |
toffset | where to begin looking in this string. | int |
Return Value: true if the character sequence represented by the argument is a prefix of the substring of this object starting at index toffset; false otherwise. The result is false if toffset is negative or greater than the length of this String object; otherwise the result is the same as the result of the expression.
this.substring(toffset).startsWith(prefix)
Return Value Type: boolean
Pictorial presentation of Java String split() Method
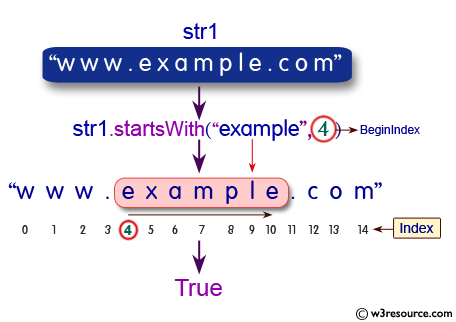
Example: Java String startsWith(String prefix, int toffset) Method
The following example shows the usage of java String() method.
import java.lang.*;
public class StringExample {
public static void main(String[] args) {
System.out.println();
String str = "www.example.com";
System.out.println(str);
// the start string to be checked
String startstr = "example";
String startstr1 = "example";
// checks that string starts with given substring and starting index
boolean returnval1 = str.startsWith(startstr);
boolean returnval2 = str.startsWith(startstr1, 4);
// prints true if the string starts with given substring
System.out.println("starts with " + startstr + " ? " + returnval1);
System.out.println("string " + startstr1 + " starting from index 4 ? "
+ returnval2);
System.out.println();
}
}
Output:
www.example.com starts with example ? false string example starting from index 4 ? true
public boolean startsWith(String prefix)
Tests if this string starts with the specified prefix.
Java Platform: Java SE 8
Syntax:
startsWith(String prefix)
Parameters:
Name | Description | Type |
---|---|---|
prefix | the prefix. | String |
Return Value: true if the character sequence represented by the argument is a prefix of the character sequence represented by this string; false otherwise. Note also that true will be returned if the argument is an empty string or is equal to this String object as determined by the equals(Object) method.
Return Value Type: boolean
Example: Java String startsWith(String prefix) Method
The following example shows the usage of java String() method.
public class Main {
public static void main(String[] args) {
System.out.println();
String str = "www.example.com";
System.out.println(str);
String startstr = "www";
String startstr1 = "http://";
boolean returnval1 = str.startsWith(startstr);
boolean returnval2 = str.startsWith(startstr1);
System.out.println(returnval1);
System.out.println(returnval2);
System.out.println();
}
}
Output:
www.example.com true false
Java Code Editor:
Previous:split Method
Next:subSequence Method
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics