Java ArrayDeque Class: getFirst() Method
public E getFirst()
The getFirst() method is used to retrieve the first element of a given deque.
This method differs from peekFirst only in that it throws an exception if this deque is empty.
Package: java.util
Java Platform: Java SE 8
Syntax:
getFirst()
Return Value:
the head of this deque
Return Value Type: E - the type of elements held in this collection
Throws:
NoSuchElementException - if this deque is empty
Pictorial Presentation
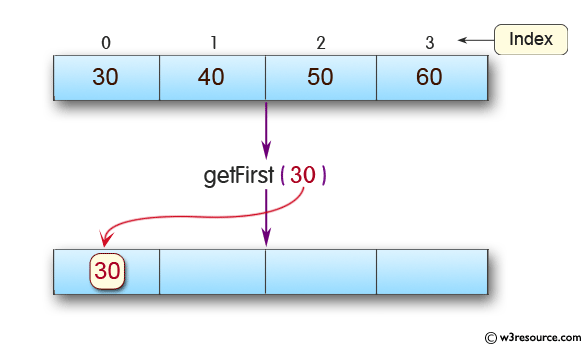
Example: Java ArrayDeque Class: getFirst() Method
import java.util.ArrayDeque;
import java.util.Deque;
public class Main {
public static void main(String[] args) {
// Create an empty array deque with an initial capacity
Deque<Integer> deque = new ArrayDeque(8);
// Use add() method to add elements in the deque
deque.add(10);
deque.add(20);
deque.add(30);
deque.add(40);
// Print all the elements of the original deque
System.out.println("Original elements of the deque: ");
for (Integer n : deque) {
System.out.println(n);
}
// Retrieve the first element of the deque.
int e = deque.getFirst();
System.out.println("Retrieve the first element: " + e);
}
}
Output:
Powered by Original elements of the deque: 10 20 30 40 Retrieve the first element: 10
Java Code Editor:
Previous: element Method
Next: getLast Method