Java ArrayDeque Class: offerLast() Method
public boolean offerLast(E e)
The offerLast() method is used to insert the specified element at the end of a given deque.
Package: java.util
Java Platform: Java SE 8
Syntax:
offerLast(E e)
Parameters:
Name | Description |
---|---|
e | the element to add |
Return Value:
true (as specified by Deque.offerLast(E))
Return Value Type: boolean
Throws:
NullPointerException - if the specified element is null
Pictorial Presentation
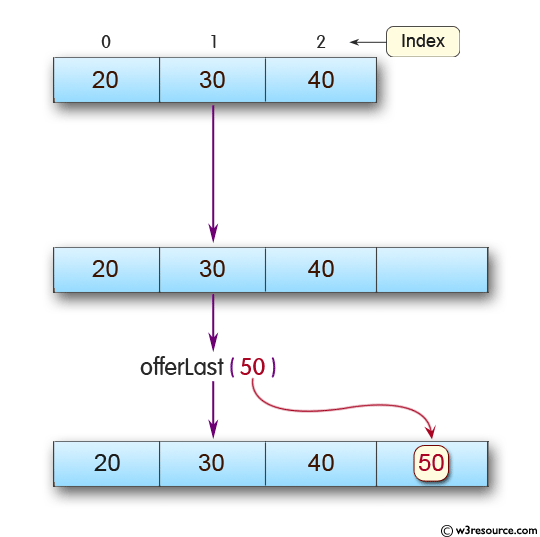
Example: Java ArrayDeque Class: offerLast() Method
import java.util.ArrayDeque;
import java.util.Deque;
public class Main {
public static void main(String[] args) {
// Create an empty array deque
Deque<Integer> deque = new ArrayDeque<Integer>(8);
// Use add() method to add elements in the deque
deque.add(20);
deque.add(30);
deque.add(40);
deque.add(35);
// Print all the elements of the original deque
System.out.println("Elements of the original deque:");
for (Integer n : deque) {
System.out.println(n);
}
// Insert 50 at the end of the deque using offer() method.
deque.offerLast(50);
// Print all the elements available in deque
System.out.println("Elements of the deque after insert an element at the last position of the deque:");
for (Integer n : deque) {
System.out.println(n);
}
}
}
Output:
Elements of the original deque: 20 30 40 35 Elements of the deque after insert an element at the last position of the deque: 20 30 40 35 50
Example of Throws: Java ArrayDeque class: offerLast() Method
NullPointerException - if the specified element is null
Let
in the above example.
Output:
Java Code Editor:
Previous:offerFirst Method
Next:peek Method