JavaScript: Parse a HTTP Cookie header string and return an object of all cookie name-value pairs
JavaScript fundamental (ES6 Syntax): Exercise-171 with Solution
Parse Cookie Header to Object
Write a JavaScript program to parse a HTTP Cookie header string and return an object of all cookie name-value pairs.
- Use String.prototype.split(';') to separate key-value pairs from each other.
- Use Array.prototype.map() and String.prototype.split('=') to separate keys from values in each pair.
- Use Array.prototype.reduce() and decodeURIComponent() to create an object with all key-value pairs.
Sample Solution:
JavaScript Code:
// Define a function 'parseCookie' that takes a string representing a cookie and parses it into an object
const parseCookie = str =>
// Split the input string into an array of key-value pairs separated by ';'
str
.split(';')
// Map over the array of key-value pairs and split each pair into an array of key and value
.map(v => v.split('='))
// Reduce the array of key-value arrays into an object
.reduce((acc, v) => {
// Decode and trim the key and value, then assign them as properties to the accumulator object
acc[decodeURIComponent(v[0].trim())] = decodeURIComponent(v[1].trim());
return acc;
}, {});
// Call the 'parseCookie' function with a sample cookie string and log the result
console.log(parseCookie('foo=bar; equation=E%3Dmc%5E2'));
Output:
{"foo":"bar","equation":"E=mc^2"}
Visual Presentation:
Flowchart:
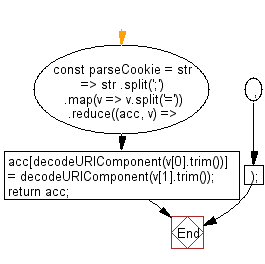
Live Demo:
See the Pen javascript-basic-exercise-171-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a function that invokes fn with partials prepended to the arguments it receives.
Next: Write a JavaScript program to create a function that invokes the provided function with its arguments transformed.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-171.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics