JavaScript: Chunk an array into smaller arrays of a specified size
JavaScript fundamental (ES6 Syntax): Exercise-265 with Solution
Chunk Array
Write a JavaScript program to chunk an array into smaller arrays of a specified size.
- Use Array.from() to create a new array, that fits the number of chunks that will be produced.
- Use Array.prototype.slice() to map each element of the new array to a chunk the length of size.
- If the original array can't be split evenly, the final chunk will contain the remaining elements.
Sample Solution:
JavaScript Code:
// Define a function 'chunk' to split an array into smaller chunks of a specified size
const chunk = (arr, size) =>
// Use Array.from to create a new array with a length equal to the number of chunks needed
Array.from({ length: Math.ceil(arr.length / size) }, (v, i) =>
// For each element in the new array, slice the original array to extract a chunk of 'size' elements
arr.slice(i * size, i * size + size)
);
// Test the function with a sample array and chunk size
console.log(chunk([1, 2, 3, 4, 5], 2)); // Output: [[1, 2], [3, 4], [5]]
Output:
[[1,2],[3,4],[5]]
Visual Presentation:
Flowchart:
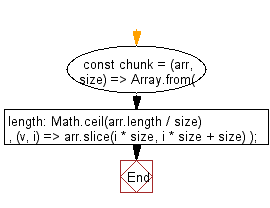
Live Demo:
See the Pen javascript-basic-exercise-265-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that splits an array into smaller arrays (chunks) of a specified size.
- Write a JavaScript function that uses a loop to divide an array into subarrays of equal length.
- Write a JavaScript program that implements array chunking without using external libraries.
- Write a JavaScript function that handles cases where the array cannot be evenly divided and returns the remaining elements in the last chunk.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to capitalize the first letter of every word in a string.
Next: Write a JavaScript program to clamp a number within the inclusive range specified by the given boundary values a and b.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.