JavaScript: Measure the time taken by a function to execute
JavaScript fundamental (ES6 Syntax): Exercise-28 with Solution
Measure Function Execution Time
Write a JavaScript program to measure the time a function to execute.
- Use Console.time() and Console.timeEnd() to measure the difference between the start and end times to determine how long the callback took to execute.
Sample Solution:
JavaScript Code:
// Define a function called `time_taken` that calculates the time taken for a given callback function to execute.
// It takes a callback function as an argument and returns the result of the callback.
const time_taken = callback => {
const result = callback(); // Execute the callback function and store the result
return result; // Return the result
};
// Example usage with different callback functions
console.log("Time taken: " + time_taken(() => Math.pow(2, 10))+" ms");
console.log("Time taken: " + time_taken(() => Math.sqrt(225))+" ms");
console.log("Time taken: " + time_taken(() => Math.sqrt((5 * 5) + (6 * 6)))+" ms");
Output:
Time taken: 1024 ms Time taken: 15 ms Time taken: 7.810249675906654 ms
Flowchart:
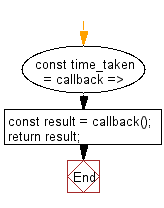
Note : The code above is written in ES6 and can be executed as intended on Chrome Developer Tools.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that measures the execution time of a given function using performance.now().
- Write a JavaScript function that wraps another function and logs the time taken to execute it.
- Write a JavaScript program that compares the execution times of two different functions and outputs the faster one.
Go to:
PREV : Find Common Elements with Comparator.
NEXT : Convert Value to Safe Integer.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.