JavaScript: Convert a value to a safe integer
JavaScript fundamental (ES6 Syntax): Exercise-29 with Solution
Convert Value to Safe Integer
Write a JavaScript program to convert a value to a safe integer.
- Use Math.max() and Math.min() to find the closest safe value.
- Use Math.round() to convert to an integer.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function named to_Safe_Integer that converts a given number to a safe integer.
const to_Safe_Integer = num =>
Math.round(Math.max(Math.min(num, Number.MAX_SAFE_INTEGER), Number.MIN_SAFE_INTEGER));
// Test the function with different input values.
console.log(to_Safe_Integer('5.2')); // Output: 5
console.log(to_Safe_Integer('5.52')); // Output: 6
console.log(to_Safe_Integer(Infinity)); // Output: 9007199254740991 (MAX_SAFE_INTEGER)
Output:
5 6 9007199254740991
Flowchart:
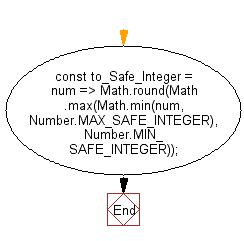
Live Demo:
See the Pen javascript-basic-exercise-1-29 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts a value to a safe integer using Number.isSafeInteger for validation.
- Write a JavaScript function that sanitizes input values and returns a safe integer or a default value if conversion fails.
- Write a JavaScript program that checks a value’s type and converts it to a safe integer using bitwise operators.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to measure the time taken by a function to execute.
Next: Write a JavaScript program to filter out the element(s) of an given array, that have one of the specified values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.