JavaScript: Check whether all elements in a given array are equal or not
JavaScript fundamental (ES6 Syntax): Exercise-56 with Solution
Check All Elements Equal in Array
Write a JavaScript program to check whether all elements in a given array are equal or not.
- Use Array.prototype.every() to check if all the elements of the array are the same as the first one.
- Elements in the array are compared using the strict comparison operator, which does not account for NaN self-inequality.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'allEqual' to check if all elements in the array are equal.
const allEqual = arr =>
// Use the 'every' method to check if all elements in the array are equal to the first element.
arr.every(val => val === arr[0]);
// Test cases
console.log(allEqual([1, 2, 3, 4, 5, 6])); // Output: false (not all elements are equal)
console.log(allEqual([12, 12, 12, 12])); // Output: true (all elements are equal)
Output:
false true
Visual Presentation:
Flowchart:
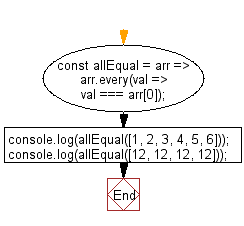
Live Demo:
See the Pen javascript-basic-exercise-56-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if every element in an array is identical.
- Write a JavaScript function that compares each array element with the first element and returns a boolean result.
- Write a JavaScript program that checks an array’s homogeneity by using the Array.every() method.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to Join all given URL segments together, then normalizes the resulting URL.
Next: Write a JavaScript program to compute the average of an array, after mapping each element to a value using the provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.