JavaScript: Compute the average of an array, after mapping each element to a value using the provided function
JavaScript fundamental (ES6 Syntax): Exercise-57 with Solution
Average of Array with Mapping Function
Write a JavaScript program to compute the average of an array, after mapping each element to a value using the provided function.
- Use Array.prototype.map() to map each element to the value returned by fn.
- Use Array.prototype.reduce() to add each value to an accumulator, initialized with a value of 0.
- Divide the resulting array by its length.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'averageBy' to calculate the average value of an array based on a given function or property.
const averageBy = (arr, fn) =>
// Map each element of the array to the value returned by the function or the value of the property.
arr.map(typeof fn === 'function' ? fn : val => val[fn])
// Calculate the sum of all values using the 'reduce' method.
.reduce((acc, val) => acc + val, 0) /
// Divide the sum by the length of the array to get the average.
arr.length;
// Test cases
console.log(averageBy([{ a: 40 }, { a: 20 }, { a: 80 }, { a: 60 }], o => o.a)); // Output: 50 (average of 'a' property)
console.log(averageBy([{ a: 4 }, { a: 2 }, { a: 8 }, { a: 6 }], 'a')); // Output: 5 (average of 'a' property)
Output:
50 5
Visual Presentation:
Flowchart:
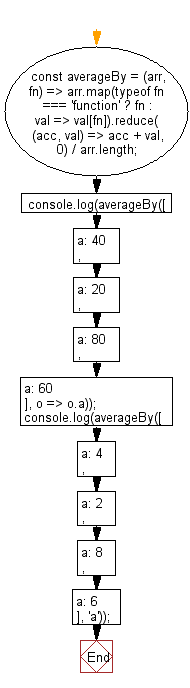
Live Demo:
See the Pen javascript-basic-exercise-57-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that computes the average of an array after transforming each element with a given function.
- Write a JavaScript function that maps each value of an array to a new value and then calculates the mean of the resulting numbers.
- Write a JavaScript program that validates and maps array elements before computing their arithmetic mean.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if all elements in a given array are equal or not.
Next: Write a JavaScript program to split values into two groups according to a predicate function, which specifies which group an element in the input collection belongs to.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.